用MapReduce统计Hbase表单科排名的代码
时间: 2023-08-31 17:24:31 浏览: 128
### 回答1:
这是一个使用 MapReduce 统计 HBase 表中科目排名的示例代码:
```
import java.io.IOException;
import java.util.StringTokenizer;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.io.ImmutableBytesWritable;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.hbase.mapreduce.TableMapper;
import org.apache.hadoop.hbase.mapreduce.TableReducer;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
public class SubjectRank {
static class SubjectMapper extends TableMapper<Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text subject = new Text();
public void map(ImmutableBytesWritable row, Result value, Context context) throws IOException, InterruptedException {
String subjectStr = new String(value.getValue(Bytes.toBytes("grades"), Bytes.toBytes("subject")));
subject.set(subjectStr);
context.write(subject, one);
}
}
static class SubjectReducer extends TableReducer<Text, IntWritable, ImmutableBytesWritable> {
public void reduce(Text subject, Iterable<IntWritable> counts, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable count : counts) {
sum += count.get();
}
Put put = new Put(Bytes.toBytes(subject.toString()));
put.addColumn(Bytes.toBytes("rank"), Bytes.toBytes("count"), Bytes.toBytes(sum));
context.write(null, put);
}
}
public static void main(String[] args) throws Exception {
Configuration conf = HBaseConfiguration.create();
Job job = Job.getInstance(conf, "subject rank");
job.setJarByClass(SubjectRank.class);
Scan scan = new Scan();
scan.addColumn(Bytes.toBytes("grades"), Bytes.toBytes("subject"));
TableMapReduceUtil.initTableMapperJob("grades", scan, SubjectMapper.class, Text.class, IntWritable.class, job);
TableMapReduceUtil.initTableReducerJob("subject_rank", SubjectReducer.class, job);
job.waitForCompletion(true);
}
}
```
这段代码
### 回答2:
使用MapReduce统计HBase表单科排名的代码通常包括以下几个步骤:
1. 创建HBase表单科数据的Mapper类。
```java
public class SpecialtyMapper extends TableMapper<Text, IntWritable> {
private Text outputKey = new Text();
private IntWritable outputValue = new IntWritable();
@Override
protected void map(ImmutableBytesWritable key, Result value, Context context) throws IOException, InterruptedException {
// 从HBase表中获取学生的单科成绩信息
String subject = Bytes.toString(key.get());
int score = Bytes.toInt(value.getValue(Bytes.toBytes("cf"), Bytes.toBytes("score")));
// 设置Mapper输出的Key为学科名称,Value为学生成绩
outputKey.set(subject);
outputValue.set(score);
context.write(outputKey, outputValue);
}
}
```
2. 创建HBase表单科排名的Reducer类。
```java
public class SpecialtyReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
private List<Pair<Integer, Text>> scores = new ArrayList<>();
@Override
protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
int count = 0;
// 统计每个学科的总成绩和人数
for (IntWritable value : values) {
sum += value.get();
count++;
}
// 将学科总成绩与学科名称保存到List中
scores.add(new Pair<>(sum, key));
}
@Override
protected void cleanup(Context context) throws IOException, InterruptedException {
// 对学科总成绩进行排序
scores.sort(Comparator.reverseOrder());
// 输出学科排名结果
int rank = 1;
for (Pair<Integer, Text> score : scores) {
context.write(score.getValue(), new IntWritable(rank++));
}
}
}
```
3. 创建Main函数来配置和运行MapReduce任务。
```java
public class SpecialtyRanking {
public static void main(String[] args) throws Exception {
Configuration conf = HBaseConfiguration.create();
Job job = Job.getInstance(conf, "Specialty Ranking");
job.setJarByClass(SpecialtyRanking.class);
// 配置Mapper和Reducer类
job.setMapperClass(SpecialtyMapper.class);
job.setReducerClass(SpecialtyReducer.class);
// 配置输入和输出的HBase表
TableMapReduceUtil.initTableMapperJob("YourHBaseTableName", new Scan(), SpecialtyMapper.class, Text.class, IntWritable.class, job);
TableMapReduceUtil.initTableReducerJob("YourOutputTableName", SpecialtyReducer.class, job);
// 设置最终输出的Key和Value类型
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上是一个简单的使用MapReduce统计HBase表单科排名的代码示例,可以根据具体需求进行适当的修改和扩展。
### 回答3:
使用MapReduce统计Hbase表单科排名的代码大致可以分为以下几个步骤:
1. 创建一个Hadoop MapReduce Job并设置相应的配置信息。
2. 实现Mapper类,用于读取Hbase表并提取所需的数据进行初步处理。
3. 实现Reducer类,用于对Mapper输出的数据进行二次处理并得出结果。
4. 在主程序中组织Mapper和Reducer,并指定输入输出路径。
5. 运行MapReduce Job并等待结果。
下面是一个示例代码的伪代码版本,具体细节需要根据实际情况做相应调整:
```java
// 导入所需的包
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.io.ImmutableBytesWritable;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
public class HBaseMapReduce {
// Mapper类
public static class HBaseMapper extends Mapper<ImmutableBytesWritable, Result, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text subject = new Text();
public void map(ImmutableBytesWritable key, Result value, Context context) throws IOException, InterruptedException {
// 从Hbase表中获取所需的数据,例如学科信息和成绩
String subjectStr = Bytes.toString(value.getValue(Bytes.toBytes("cf"), Bytes.toBytes("subject")));
int score = Bytes.toInt(value.getValue(Bytes.toBytes("cf"), Bytes.toBytes("score")));
// 输出学科和成绩,作为Reducer的输入
subject.set(subjectStr);
context.write(subject, one);
}
}
// Reducer类
public static class HBaseReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
// 对统计结果进行累加求和
for (IntWritable value : values) {
sum += value.get();
}
// 输出学科和总分,作为最终结果
context.write(key, new IntWritable(sum));
}
}
public static void main(String[] args) throws Exception {
// 创建Hadoop MapReduce Job并设置配置信息
Configuration conf = HBaseConfiguration.create();
Job job = Job.getInstance(conf, "HBase MapReduce");
job.setJarByClass(HBaseMapReduce.class);
// 从Hbase表中读取数据
Scan scan = new Scan();
scan.setCaching(500);
scan.setCacheBlocks(false);
TableMapReduceUtil.initTableMapperJob("tablename", scan, HBaseMapper.class, Text.class, IntWritable.class, job);
// 指定Reducer类,并设置最终的输出路径
job.setReducerClass(HBaseReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileOutputFormat.setOutputPath(job, new Path("output"));
// 运行MapReduce Job并等待结果
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
这个示例代码中,假设Hbase表中包含"tablename"表,具有"subject"和"score"两列。代码通过Mapper将学科和成绩作为键值对输出,在Reducer中对学科的成绩进行累加求和,并将结果输出到指定的输出路径中。请根据实际情况对代码进行相应调整,并确保相关依赖包的正确导入。
阅读全文
相关推荐
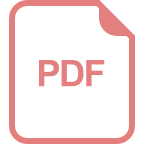
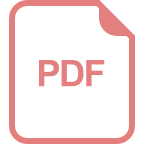














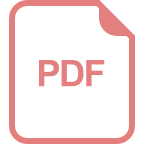
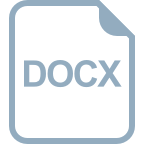
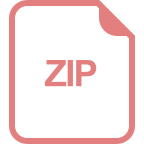