用MapReduce排序Hbase表中的成绩的代码
时间: 2023-02-05 10:44:11 浏览: 57
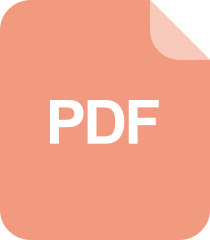
通用MapReduce程序复制HBase表数据
下面是用 MapReduce 排序 Hbase 表中的成绩的示例代码:
```
import java.io.IOException;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Put;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.io.ImmutableBytesWritable;
import org.apache.hadoop.hbase.mapreduce.TableMapReduceUtil;
import org.apache.hadoop.hbase.mapreduce.TableMapper;
import org.apache.hadoop.hbase.mapreduce.TableReducer;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
public class HBaseSort {
public static class HBaseMapper extends TableMapper<Text, IntWritable> {
public static final byte[] CF = "cf".getBytes();
public static final byte[] ATTR1 = "grade".getBytes();
private final IntWritable grade = new IntWritable();
private final Text row = new Text();
@Override
public void map(ImmutableBytesWritable row, Result value, Context context) throws IOException, InterruptedException {
// 获取行键
String rowKey = new String(row.get());
this.row.set(rowKey);
// 获取成绩
byte[] bGrade = value.getValue(CF, ATTR1);
int intGrade = Bytes.toInt(bGrade);
grade.set(intGrade);
// 输出行键和成绩
context.write(row, grade);
}
}
public static class HBaseReducer extends TableReducer<Text, IntWritable, ImmutableBytesWritable> {
public static final byte[] CF = "cf".getBytes();
public static final byte[] ATTR1 = "grade".getBytes();
@Override
public void reduce(Text row, Iterable<IntWritable> grades, Context context) throws IOException, InterruptedException {
// 遍历成绩列表,将成绩写入 HBase 表
for (IntWritable grade : grades) {
Put put = new Put(Bytes.toBytes(row.toString()));
put.addColumn(CF, ATTR1, Bytes.toBytes(grade.get()));
context.write(new ImmutableBytesWritable(Bytes.toBytes(row.toString())), put);
}
}
}
public static void main(String[] args) throws Exception {
Configuration
阅读全文
相关推荐
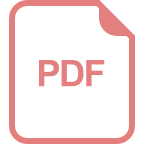
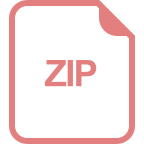







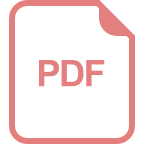
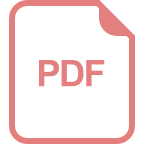
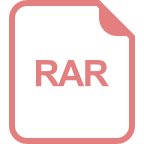
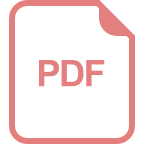
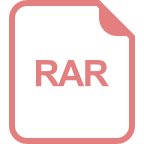
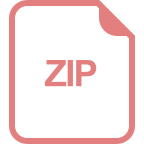