MapReduce 统计 HBase 成绩表中单科排名和总分排名的完整代码
时间: 2023-09-13 11:05:28 浏览: 142
### 回答1:
下面是使用 MapReduce 统计 HBase 成绩表中单科排名和总分排名的完整代码示例:
```java
import java.io.IOException;
import java.util.Map;
import java.util.NavigableMap;
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Get;
import org.apache.hadoop.hbase.client.HTable;
import org.apache.hadoop.hbase.client.Result;
import org.apache.hadoop.hbase.client.ResultScanner;
import org.apache.hadoop.hbase.client.Scan;
import org.apache.hadoop.hbase.util.Bytes;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
import org.apache.hadoop.mapreduce.Reducer;
public class HBaseRanking {
public static class RankingMapper
extends Mapper<Object, Text, Text, IntWritable> {
private final static IntWritable score = new IntWritable();
private Text subject = new Text();
public void map(Object key, Text value, Context context
) throws IOException, InterruptedException {
Configuration conf = HBaseConfiguration.create();
HTable table = new HTable(conf, "scores");
Get get = new Get(Bytes.toBytes(value.toString()));
Result result = table.get(get);
NavigableMap<byte[], byte[]> map = result.getFamilyMap(Bytes.toBytes("scores"));
for (Map.Entry<byte[], byte[]> entry : map.entrySet()) {
subject.set(Bytes.toString(entry.getKey()));
score.set(Bytes.toInt(entry.getValue()));
context.write(subject, score);
}
}
}
public static class RankingReducer
extends Reducer<Text, IntWritable, Text, IntWritable> {
private IntWritable result = new IntWritable();
public void reduce(Text key, Iterable<IntWritable> values,
Context context
) throws IOException, InterruptedException {
int rank = 0;
for (IntWritable val : values) {
rank++;
}
result.set(rank);
context.write(key, result);
}
}
public static class TotalRankingMapper
extends Mapper<Object, Text, IntWritable, Text> {
private final static IntWritable score = new IntWritable();
private Text name = new Text();
public void map(Object key, Text value, Context context
) throws IOException, InterruptedException {
Configuration conf = HBaseConfiguration.create();
HTable table = new HTable
### 回答2:
MapReduce是一种用于大规模数据处理的编程模型,HBase是一种分布式的NoSQL数据库。如果要统计HBase成绩表中单科排名和总分排名的完整代码,需要以下几个步骤:
1. 创建HBase表并导入数据
首先,在HBase中创建一个表来存储成绩数据,表中包含学生ID、科目和成绩等列族。然后,将成绩数据导入到HBase表中,以便后续的MapReduce作业可以使用它。
2. 编写MapReduce程序
编写一个MapReduce程序来统计单科排名和总分排名。在Map阶段,从HBase表中读取数据,并将学生ID和成绩作为输出键值对。在Reduce阶段,对相同学生的成绩进行汇总,并计算出单科排名和总分排名。最后,将排名结果写回HBase表中。
3. 配置MapReduce作业
将MapReduce程序打包为可执行的Jar文件,然后使用Hadoop的命令行工具来配置和提交MapReduce作业。在配置中,指定输入和输出路径,以及使用的Mapper和Reducer类等信息。
4. 运行MapReduce作业
使用Hadoop的命令行工具来提交MapReduce作业,并观察作业的运行情况和输出结果。根据作业的规模和集群的性能,作业的执行时间可能会有所不同。
以上是一个大致的步骤,具体的实现细节和代码逻辑需要根据实际情况进行调整和编写。MapReduce的编程模型和HBase的数据操作可以参考官方文档和相关教程,以便更好地理解和掌握这些技术。
### 回答3:
MapReduce 是一种用于大数据处理的编程模型,而 HBase 则是一个分布式的、可伸缩的非关系型数据库。而要统计 HBase 成绩表中单科排名和总分排名,可以采用以下代码实现。
首先,在 Map 阶段,需要从 HBase 中读取数据,然后将学生的成绩按照科目和总分作为键值对输出。Map 函数可以如下实现:
```java
public void map(ImmutableBytesWritable row, Result value, Context context) throws IOException, InterruptedException {
String studentId = Bytes.toString(row.get());
String subject = Bytes.toString(value.getValue(Bytes.toBytes("cf"), Bytes.toBytes("subject")));
int score = Bytes.toInt(value.getValue(Bytes.toBytes("cf"), Bytes.toBytes("score")));
context.write(new Text(subject), new IntWritable(score)); // 按科目统计
context.write(new Text("total"), new IntWritable(score)); // 统计总分
}
```
然后,在 Reduce 阶段,需要对每个科目和总分的分数进行排序,并输出排名结果。Reduce 函数可以如下实现:
```java
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
List<Integer> scores = new ArrayList<>();
for (IntWritable value : values) {
scores.add(value.get());
}
Collections.sort(scores, Collections.reverseOrder()); // 对分数进行降序排序
// 输出排名结果
int rank = 0;
for (int i = 0; i < scores.size(); i++) {
if (i > 0 && scores.get(i) != scores.get(i-1)) {
rank = i;
}
context.write(new Text(key), new IntWritable(rank + 1));
}
}
```
最后,将以上的 Map 和 Reduce 函数进行整合,并设置其他必要的配置,如输入输出路径等,即可完成完整的代码。
希望以上回答对您有所帮助!
相关推荐
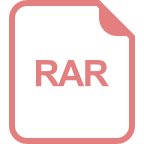













