import cv2 import pyautogui # 加载红色人物的模板图像 template = cv2.imread('template.png') # 设置阈值,将图像转换为二值图像 threshold = 0.8 # 设置鼠标移动的速度 speed = 0.5 while True: # 截取屏幕图像 screenshot = pyautogui.screenshot() # 将截图转换为OpenCV格式 screenshot = cv2.cvtColor(numpy.array(screenshot), cv2.COLOR_RGB2BGR) # 在截图中搜索红色人物 result = cv2.matchTemplate(screenshot, template, cv2.TM_CCOEFF_NORMED) locations = numpy.where(result >= threshold) locations = list(zip(*locations[::-1])) # 如果找到了红色人物,则将鼠标移动到其上方 if locations: x, y = locations[0] pyautogui.moveTo(x + template.shape[1] / 2, y + template.shape[0] / 2, duration=speed)
时间: 2024-04-02 07:33:02 浏览: 166
这段代码使用了Python的OpenCV库和PyAutoGUI库,实现了一个自动寻找屏幕中指定图像并将鼠标移动到其上方的功能。具体来说,它通过读取一张名为template.png的模板图像,并使用OpenCV的matchTemplate函数在屏幕截图中搜索该图像。如果找到了模板图像,则获取其位置并使用PyAutoGUI的moveTo函数将鼠标移动到该位置上方。其中,threshold参数设置了匹配的阈值,speed参数设置了鼠标移动的速度。这个程序可以用于一些简单的自动化任务,比如自动点击某个按钮或者自动填写某个表单。
相关问题
import cv2 import numpy as np import pyautogui import tkinter as tk from PIL import ImageTk, Image class WindowDetector: def __init__(self, template_path): self.template = cv2.imread(template_path, 0) self.w, self.h = self.template.shape[::-1] def detect(self): screenshot = pyautogui.screenshot() screenshot = np.array(screenshot) screenshot = cv2.cvtColor(screenshot, cv2.COLOR_BGR2GRAY) res = cv2.matchTemplate(screenshot, self.template, cv2.TM_CCOEFF_NORMED) threshold = 0.8 loc = np.where(res >= threshold) for pt in zip(*loc[::-1]): cv2.rectangle(screenshot, pt, (pt[0] + self.w, pt[1] + self.h), (0, 0, 255), 2) return screenshot class App: def __init__(self, template_path): self.window_detector = WindowDetector(template_path) self.root = tk.Tk() self.root.title("Window Detector") self.root.geometry("800x600") self.canvas = tk.Canvas(self.root, width=800, height=600) self.canvas.pack() self.template = ImageTk.PhotoImage(Image.open(template_path)) tk.Button(self.root, text="Detect", command=self.detect_window).pack() tk.Label(self.root, image=self.template).pack() self.root.mainloop() def detect_window(self): screenshot = self.window_detector.detect() img = ImageTk.PhotoImage(Image.fromarray(screenshot)) self.canvas.create_image(0, 0, anchor=tk.NW, image=img) self.root.update_idletasks() if __name__ == "__main__": app = App("template.png")
这段代码是用来检测屏幕上是否存在一个特定的窗口,它首先读取一个模板图像,然后使用pyautogui库截取屏幕图像。接着,它使用OpenCV中的模板匹配算法来在屏幕截图中寻找模板图像的匹配位置。如果匹配程度超过了设定的阈值,就会在屏幕截图中标出匹配的位置。最后,它使用tkinter库创建了一个GUI窗口,其中包含一个按钮和一个标签,用户可以点击按钮来检测屏幕上是否存在指定的窗口,并在标签中显示检测结果。
又报错了,cv2.error: OpenCV(4.7.0) D:\a\opencv-python\opencv-python\opencv\modules\imgproc\src\templmatch.cpp:588: error: (-215:Assertion failed) corr.rows <= img.rows + templ.rows - 1 && corr.cols <= img.cols + templ.cols - 1 in function 'cv::crossCorr'
这个错误通常是由于模板的大小超过待匹配图像的大小所引起的。请确保你的模板图片的尺寸不要超过游戏界面截图的尺寸。
如果你的模板图片尺寸确实超出了游戏界面截图的尺寸,你可以考虑调整模板图片的大小,使其适应游戏界面截图的尺寸。你可以使用OpenCV中的`cv2.resize()`函数来调整图片的大小。
下面是一个示例代码,展示了如何调整模板图片的大小:
```python
import cv2
import numpy as np
# 加载游戏界面截图和图片文件
game_screen = cv2.imread('game_screen.png')
image_folder = 'image_folder/'
# 遍历图片文件夹中的所有图片
for file in os.listdir(image_folder):
if file.endswith('.png') or file.endswith('.jpg'):
# 加载当前图片
image_path = os.path.join(image_folder, file)
template = cv2.imread(image_path)
# 调整模板图片的大小以适应游戏界面截图
template = cv2.resize(template, (game_screen.shape[1], game_screen.shape[0]))
# 使用模板匹配方法查找相似区域
result = cv2.matchTemplate(game_screen, template, cv2.TM_CCOEFF_NORMED)
threshold = 0.8 # 设定阈值,可以根据具体情况调整
locations = np.where(result >= threshold)
# 标记相似区域
for loc in zip(*locations[::-1]):
top_left = loc
bottom_right = (top_left[0] + template.shape[1], top_left[1] + template.shape[0])
cv2.rectangle(game_screen, top_left, bottom_right, (0, 255, 0), 2)
# 显示画有标记的游戏界面
cv2.imshow('Game Screen', game_screen)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在这个示例代码中,我们使用`cv2.resize()`函数将模板图片调整为与游戏界面截图相同的尺寸。然后,再进行模板匹配操作。这样可以确保模板图片和游戏界面截图的尺寸一致,避免了尺寸不匹配的错误。
阅读全文
相关推荐
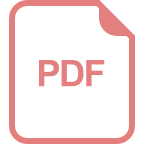
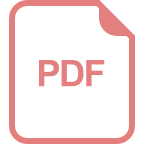
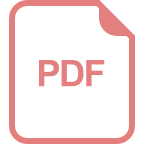
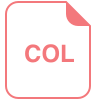



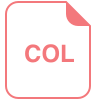
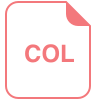
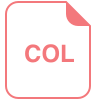
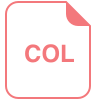
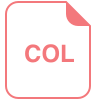
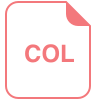
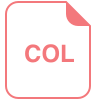
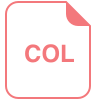
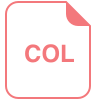
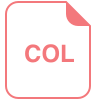