Python扫雷游戏代码
时间: 2023-09-03 11:27:37 浏览: 49
### 回答1:
在 Python 中实现扫雷游戏,需要使用到如下的基本概念和技术:
- 二维数组:可以使用列表或者 NumPy 数组来表示游戏的棋盘。
- 循环:用于遍历棋盘中的每个格子。
- 条件语句:用于判断每个格子是否是地雷。
- 函数:可以封装扫雷的相关逻辑,并且方便调用。
以下是一个简单的 Python 代码示例,实现了扫雷游戏的基本功能:
```python
import random
# 定义游戏的棋盘,其中 0 表示空格,-1 表示地雷
board = [
[0, 0, 0, 0, 0],
[0, 0, 0, 0, 0],
[0, 0, 0, 0, 0],
[0, 0, 0, 0, 0],
[0, 0, 0, 0, 0]
]
# 随机放置 10 个地雷
for i in range(10):
x = random.randint(0, 4)
y = random.randint(0, 4)
board[x][y] = -1
# 定义函数,用于扫雷
def sweep(x, y):
# 判断坐标是否越界
if x < 0 or x >= 5 or y < 0 or y >= 5:
return
# 判断坐标是否是地雷
if board[x][y] == -1:
print("Game Over!")
return
# 判断坐标是否已经被扫过
if board[x][y] != 0:
return
# 标记坐标已经被扫过
board[x][y] = 1
# 递归扫周围的八个格子
sweep(x - 1, y - 1)
sweep(x - 1, y)
sweep(x - 1, y + 1)
sweep(x, y - 1)
sweep(x, y
### 回答2:
Python扫雷游戏代码的实现通常使用面向对象的方式。下面是一个简单的示例:
```python
import random
class Minesweeper:
def __init__(self, rows, cols, mines):
self.rows = rows
self.cols = cols
self.mines = mines
self.board = [[0 for _ in range(cols)] for _ in range(rows)]
self.generate_board()
def generate_board(self):
mines_placed = 0
while mines_placed < self.mines:
row = random.randint(0, self.rows - 1)
col = random.randint(0, self.cols - 1)
# 如果该位置已经有雷,跳过
if self.board[row][col] == -1:
continue
self.board[row][col] = -1
mines_placed += 1
# 为每个非雷位置计算周围雷的数量
for row in range(self.rows):
for col in range(self.cols):
if self.board[row][col] != -1:
self.board[row][col] = self.count_mines(row, col)
def count_mines(self, row, col):
count = 0
for i in range(-1, 2):
for j in range(-1, 2):
if 0 <= row + i < self.rows and 0 <= col + j < self.cols:
if self.board[row + i][col + j] == -1:
count += 1
return count
def print_board(self):
for row in self.board:
print(' '.join([str(cell) for cell in row]))
def play(self, row, col):
if self.board[row][col] == -1:
print("Game Over!")
return
elif self.board[row][col] == 0:
print("Clicked on empty space. Keep playing...")
self.show_empty_cells(row, col)
else:
print(f"Clicked on cell with {self.board[row][col]} neighboring mines.")
def show_empty_cells(self, row, col):
if self.board[row][col] != 0:
return
self.board[row][col] = -2
for i in range(-1, 2):
for j in range(-1, 2):
if 0 <= row + i < self.rows and 0 <= col + j < self.cols:
self.show_empty_cells(row + i, col + j)
# 游戏示例
game = Minesweeper(10, 10, 10)
game.print_board()
game.play(5, 5)
```
这个示例中,我们创建了一个Minesweeper类,该类包含初始化游戏棋盘的方法generate_board()和计算周围雷数量的方法count_mines()。还有一个play()方法用于处理玩家点击的逻辑。show_empty_cells()方法用于展示周围空格的逻辑。
你可以根据需要修改这个示例代码来增加游戏界面、计时器、点击事件处理等功能。
相关推荐
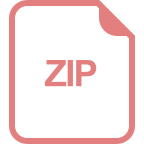
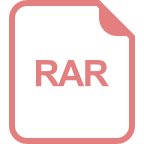
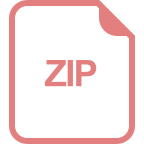










