用c语言的尾插法创建一个结点数据元素为字符型的链表,将数据元素输出
时间: 2024-09-25 22:13:01 浏览: 18
在C语言中,尾插法是一种在链表的最后添加新节点的方法。假设我们有一个结构体`Node`表示链表节点,包含一个字符型的数据成员`data`和指向下一个节点的指针`next`:
```c
typedef struct Node {
char data;
struct Node* next;
} Node;
void tailInsert(Node** head, char value) {
// 如果链表为空,直接插入新节点
if (*head == NULL) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
*head = newNode;
} else {
Node* current = *head; // 从头开始遍历
while (current->next != NULL) {
current = current->next;
}
// 在最后一个节点之后插入新节点
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
current->next = newNode;
}
}
// 输出链表中的所有字符
void printList(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%c ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
Node* head = NULL;
tailInsert(&head, 'A'); // 插入第一个字符 'A'
tailInsert(&head, 'B');
tailInsert(&head, 'C');
printList(head); // 输出链表:A B C
return 0;
}
```
在这个例子中,`tailInsert`函数用于尾部插入字符,而`printList`函数则用于遍历并打印链表中的数据。在`main`函数中创建了三个字符节点,并显示了它们。
相关推荐
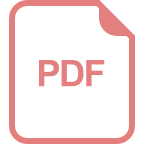
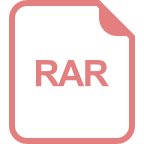
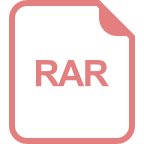













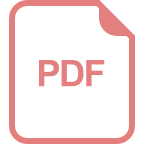
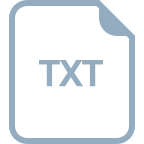