用C语言创建头插法创建一个结点数据元素为字符型的链表,将数据元素输出
时间: 2024-09-25 16:13:21 浏览: 17
在C语言中,我们可以使用结构体来表示链表节点,每个节点包含一个字符型的数据元素和指向下一个节点的指针。首先,我们需要定义一个名为`node`的结构体:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
char data; // 数据元素,字符类型
struct Node* next; // 指向下一个节点的指针
} Node;
// 创建新的链表节点函数
Node* create_new_node(char data) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (newNode != NULL) {
newNode->data = data;
newNode->next = NULL;
return newNode;
}
printf("Memory allocation failed.\n");
return NULL;
}
// 插入头节点的函数
void insert_at_head(Node** head, char data) {
Node* newNode = create_new_node(data);
if (newNode != NULL) {
newNode->next = *head;
*head = newNode;
}
}
// 打印链表中所有节点数据的函数
void print_list(Node* head) {
while (head != NULL) {
printf("%c -> ", head->data);
head = head->next;
}
printf("NULL\n");
}
int main() {
Node* head = NULL; // 初始化链表头指针
// 插入一些字符到链表头部
insert_at_head(&head, 'A');
insert_at_head(&head, 'B');
insert_at_head(&head, 'C');
// 输出链表内容
print_list(head);
return 0;
}
```
在这个例子中,我们首先创建了一个`Node`结构体,然后定义了插入新节点和打印链表的函数。在`main`函数中,我们初始化链表头指针并插入一些字符,最后打印出链表的内容。
相关推荐
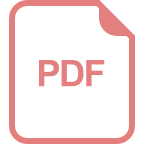
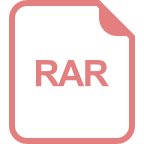
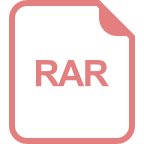













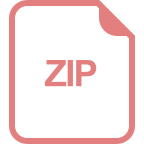
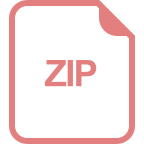