flink实时获取mysql表结构
时间: 2023-10-16 12:57:38 浏览: 61
您可以使用Flink的JDBC连接器来实时获取MySQL表的结构。以下是一个简单的代码示例:
```java
import org.apache.flink.api.common.typeinfo.TypeInformation;
import org.apache.flink.api.java.tuple.Tuple2;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.table.api.EnvironmentSettings;
import org.apache.flink.table.api.Table;
import org.apache.flink.table.api.bridge.java.StreamTableEnvironment;
import org.apache.flink.table.sources.tsextractors.ExistingField;
import org.apache.flink.table.sources.wmstrategies.AscendingTimestamps;
import org.apache.flink.table.sources.wmstrategies.BoundedOutOfOrderTimestamps;
import org.apache.flink.table.sources.wmstrategies.PunctuatedWatermarks;
import org.apache.flink.table.sources.wmstrategies.WatermarkStrategy;
import org.apache.flink.types.Row;
public class FlinkMySQLTableSchema {
public static void main(String[] args) throws Exception {
// 创建流式执行环境
StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
EnvironmentSettings settings = EnvironmentSettings.newInstance().useBlinkPlanner().inStreamingMode().build();
StreamTableEnvironment tableEnv = StreamTableEnvironment.create(env, settings);
// 定义MySQL连接器
tableEnv.executeSql("CREATE TABLE mysql_table (\n" +
" id INT,\n" +
" name STRING,\n" +
" age INT\n" +
") WITH (\n" +
" 'connector' = 'jdbc',\n" +
" 'url' = 'jdbc:mysql://localhost:3306/test',\n" +
" 'table-name' = 'your_table_name',\n" +
" 'username' = 'your_username',\n" +
" 'password' = 'your_password',\n" +
" 'lookup.cache.max-rows' = '500'\n" +
")");
// 获取表结构
Table table = tableEnv.from("mysql_table");
TypeInformation<Row> typeInfo = table.getSchema().toRowType();
String[] fieldNames = table.getSchema().getFieldNames();
TypeInformation<?>[] fieldTypes = table.getSchema().getFieldTypes();
// 打印表结构
for (int i = 0; i < fieldNames.length; i++) {
System.out.println("Field Name: " + fieldNames[i] + ", Field Type: " + fieldTypes[i]);
}
// 执行作业
env.execute();
}
}
```
您需要根据实际情况修改代码中的MySQL连接信息和表名,然后运行代码即可实时获取MySQL表的结构。请确保您已经将Flink的相关依赖添加到项目中。
相关推荐
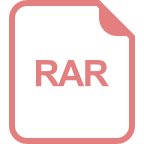














