import numpy as np import matplotlib.pyplot as plt plt.rcParams["font.sans-serif"]=["SimHei"] plt.rcParams["axes.unicode_minus"]=False data=np.random.randint(40,101,(1000,)) bins=[40,60,70,80,90,100] c1,c2,c3,c4,c5 = plt.hist( data, bins=bins, align="mid", histtype='bar', rwidth=0.3, color='r', hatch='/',) h_1 = c1.sum() plt.text(50,h_1,s='不及格:'+'{}'.format(h_1),ha='center') h_2 = c2.sum() plt.text(65,h_2,s='及格:'+'{}'.format(h_2),ha='center') h_3 = c3.sum() plt.text(75,h_3,s='中等:'+'{}'.format(h_3),ha='center') h_4 = c4.sum() plt.text(85,h_4,s='良好:'+'{}'.format(h_4),ha='center') h_5 = c5.sum() plt.text(95,h_4,s='优秀:'+'{}'.format(h_5),ha='center') plt.title("成绩统计直方图") plt.xlabel("成绩区间") plt.xticks(bins) plt.ylabel("数量") plt.show()报错:not enough values to unpack (expected 5, got 3)怎么修改
时间: 2023-12-27 20:03:44 浏览: 29
这个错误通常是因为 bins 参数指定的区间数量与返回值的数量不匹配导致的。
在这段代码中,bins 参数指定了 6 个区间,但是只有 3 个返回值。这是因为 hist 函数默认只返回三个值,分别是:
1. 直方图数据(频数)
2. 直方图数据的区间范围
3. 直方图的 patches 对象,用于修改直方图的外观
因此,您需要修改代码,将返回值的数量与 bins 参数的数量匹配。您可以通过指定一个 `_` 的变量名来忽略不需要的返回值,然后再将需要的数据存储到变量中,例如:
```python
freq, bins, patches = plt.hist(data, bins=bins, align="mid", ...)
c1, c2, c3, c4, c5 = freq
```
这样就可以将直方图数据的频数存储到变量 c1、c2、c3、c4、c5 中,然后按照原来的方法继续处理。
完整的修改后的代码如下:
```python
import numpy as np
import matplotlib.pyplot as plt
plt.rcParams["font.sans-serif"] = ["SimHei"]
plt.rcParams["axes.unicode_minus"] = False
data = np.random.randint(40, 101, (1000,))
bins = [40, 60, 70, 80, 90, 100]
freq, bins, patches = plt.hist(
data,
bins=bins,
align="mid",
histtype='bar',
rwidth=0.3,
color='r',
hatch='/',
)
c1, c2, c3, c4, c5 = freq
h_1 = c1.sum()
plt.text(50, h_1, s='不及格:'+'{}'.format(h_1), ha='center')
h_2 = c2.sum()
plt.text(65, h_2, s='及格:'+'{}'.format(h_2), ha='center')
h_3 = c3.sum()
plt.text(75, h_3, s='中等:'+'{}'.format(h_3), ha='center')
h_4 = c4.sum()
plt.text(85, h_4, s='良好:'+'{}'.format(h_4), ha='center')
h_5 = c5.sum()
plt.text(95, h_5, s='优秀:'+'{}'.format(h_5), ha='center')
plt.title("成绩统计直方图")
plt.xlabel("成绩区间")
plt.xticks(bins)
plt.ylabel("数量")
plt.show()
```
希望这可以帮助到您。
相关推荐
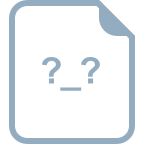
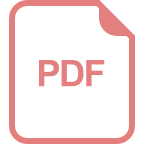
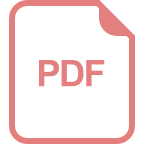















