不需要任何中文#include <stdio.h> #include <string.h> #define MAX_LEN 100 int main() { char s[MAX_LEN + 1]; char c; int len, i, j; int found = 0; printf("请输入一个字符串:"); scanf("%s", s); printf("请输入一个字符:"); scanf(" %c", &c); len = strlen(s); for (i = 0, j = 0; i < len; i++) { if (s[i] == c) { found = 1; continue; } s[j++] = s[i]; } s[j] = '\0'; if (found) { printf("删除 %c 后的字符串为:%s\n", c, s); } else { printf("NotFound\n"); } return 0; }用c语言编写,不需要中文!
时间: 2023-07-16 11:13:35 浏览: 122
以下是不需要中文的C代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
int main() {
char s[MAX_LEN + 1];
char c;
int len, i, j;
int found = 0;
printf("Please enter a string: ");
scanf("%s", s);
printf("Please enter a character: ");
scanf(" %c", &c);
len = strlen(s);
for (i = 0, j = 0; i < len; i++) {
if (s[i] == c) {
found = 1;
continue;
}
s[j++] = s[i];
}
s[j] = '\0';
if (found) {
printf("String after deleting %c: %s\n", c, s);
} else {
printf("NotFound\n");
}
return 0;
}
```
相关问题
#include <stdio.h> #include <stdlib.h> static char buff[256]; static char* string; int main() { printf("Please input your string:"); gets(string); printf("\nYour string is:%s\n",string); }
这段代码是一个简单的C程序,它的功能是从标准输入中读取一行字符串并输出。
然而,这段代码存在一个严重的问题:它使用了不安全的gets函数来读取输入,可能会导致缓冲区溢出漏洞。正确的做法是使用fgets函数来代替gets函数,限制读取的字符数量。
另外,这段代码声明了一个静态的字符数组buff,但是却没有使用它。而指针变量string却没有被初始化,使用时会导致未定义的行为。应该将string指向buff数组,或者使用动态内存分配函数malloc来为string分配内存。
改进后的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_LEN 256
int main() {
char buff[MAX_LEN];
char *string = (char*)malloc(MAX_LEN * sizeof(char));
printf("Please input your string:");
fgets(buff, MAX_LEN, stdin);
sscanf(buff, "%s", string); // 去掉字符串末尾的换行符
printf("\nYour string is:%s\n", string);
free(string);
return 0;
}
```
优化这段代码,注意 其他字符(每个中文字符算1个):#include <stdio.h> #include <ctype.h> #include <string.h> #define MAX_LEN 1000 int main() { int english = 0, digit = 0, space = 0, other = 0; char str[MAX_LEN]; fgets(str, MAX_LEN, stdin); int len = strlen(str); for (int i = 0; i < len; i++) { char c = str[i]; if (isalpha(c)) { english++; } else if (isdigit(c)) { digit++; } else if (isspace(c)) { space++; } else { other++; } } printf("%d %d %d %d", english, digit, space, other); return 0; }
可以通过以下方式来优化该代码:
1. 使用 getchar() 代替 fgets() 函数,这样可以避免不必要的内存分配和拷贝操作。
2. 不需要使用 strlen() 函数来获取字符串的长度,可以在读入字符串时同时统计字符串长度。
3. 可以将字符类型的变量 c 改为整型变量,这样可以提高运行效率。
4. 在判断字符类型时,可以使用 switch 语句代替多个 if-else 语句,这样可以提高代码可读性和运行效率。
优化后的代码如下:
```c
#include <stdio.h>
#include <ctype.h>
#define MAX_LEN 1000
int main() {
int english = 0, digit = 0, space = 0, other = 0;
int c, len = 0;
while ((c = getchar()) != EOF && len < MAX_LEN - 1) {
if (isalpha(c)) {
english++;
} else if (isdigit(c)) {
digit++;
} else if (isspace(c)) {
space++;
} else {
other++;
}
len++;
}
printf("%d %d %d %d", english, digit, space, other);
return 0;
}
```
阅读全文
相关推荐
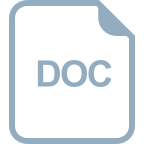
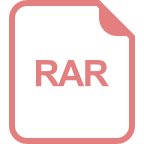














