#include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_LEN 1024 int main() { char filename[MAX_LEN]; char text[MAX_LEN]; char keyword[MAX_LEN]; int count = 0; printf("请输入文件名:"); scanf("%s", filename); FILE* outfile = fopen(filename, "w"); if (outfile == NULL) { fprintf(stderr, "无法创建文件\n"); return 1; } printf("请输入一段文字:\n"); getchar(); fgets(text, MAX_LEN, stdin); fprintf(outfile, "%s", text); fclose(outfile); printf("请输入要查找的关键字:"); scanf("%s", keyword); FILE* infile = fopen(filename, "r"); if (infile == NULL) { fprintf(stderr, "无法打开文件\n"); return 1; } char line[MAX_LEN]; while (fgets(line, MAX_LEN, infile)) { char* pos = line; while ((pos = strstr(pos, keyword))) { count++; pos++; } } printf("文件中共有 %d 个关键字。\n", count); return 0; }解释一下每一行代码的含义与用途,我看不懂
时间: 2024-01-17 18:05:05 浏览: 29
好的,我来解释一下每一行代码的含义和用途。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 1024
```
这里是引入头文件和定义常量。`stdio.h` 包含了输入输出函数,`stdlib.h` 包含了一些常用的函数,`string.h` 包含了字符串操作函数。`MAX_LEN` 是定义的一个常量,表示字符串最大长度为 1024。
```
int main() {
char filename[MAX_LEN];
char text[MAX_LEN];
char keyword[MAX_LEN];
int count = 0;
```
定义了 `main` 函数,并声明了存储文件名、文本、关键字和计数变量的数组。
```
printf("请输入文件名:");
scanf("%s", filename);
```
输出提示信息,读取用户输入的文件名。
```
FILE* outfile = fopen(filename, "w");
if (outfile == NULL) {
fprintf(stderr, "无法创建文件\n");
return 1;
}
printf("请输入一段文字:\n");
getchar();
fgets(text, MAX_LEN, stdin);
fprintf(outfile, "%s", text);
fclose(outfile);
```
使用 `fopen` 函数打开文件,并将用户输入的文本写入文件中。`getchar` 函数读取上一个输入操作中的回车符,避免在 `fgets` 函数中读取到回车符。最后关闭文件。
```
printf("请输入要查找的关键字:");
scanf("%s", keyword);
FILE* infile = fopen(filename, "r");
if (infile == NULL) {
fprintf(stderr, "无法打开文件\n");
return 1;
}
char line[MAX_LEN];
while (fgets(line, MAX_LEN, infile)) {
char* pos = line;
while ((pos = strstr(pos, keyword))) {
count++;
pos++;
}
}
printf("文件中共有 %d 个关键字。\n", count);
return 0;
}
```
打开文件并读取文件中的每一行,查找关键字并计数。最后输出关键字的数量,程序结束。
希望这些解释能够帮助你理解代码的含义和作用。
相关推荐
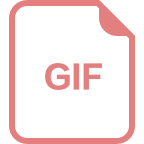
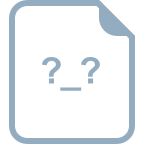
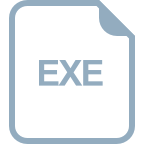















