stm32控制4*4键盘
时间: 2023-10-14 18:06:38 浏览: 69
要使用STM32控制4*4键盘,可以按照以下步骤进行:
1. 确定键盘的引脚连接方式,通常4*4键盘有8个引脚,其中4个为行(ROW)引脚,4个为列(COLUMN)引脚。
2. 将行引脚连接到STM32的GPIO引脚上,将列引脚连接到另外的GPIO引脚上。
3. 配置STM32的GPIO引脚为输入模式,以读取键盘的按键状态。
4. 循环扫描键盘的状态,对于每一个行引脚,依次将其设置为高电平,然后读取每一个列引脚的状态,判断是否有按键按下。
5. 如果检测到有按键按下,则可以根据按键的位置进行相应的操作,例如发送数据或者执行某个函数。
下面是一个简单的示例代码,用于控制4*4键盘:
```c
#include "stm32f10x.h"
#define ROW1 GPIO_Pin_0
#define ROW2 GPIO_Pin_1
#define ROW3 GPIO_Pin_2
#define ROW4 GPIO_Pin_3
#define COL1 GPIO_Pin_4
#define COL2 GPIO_Pin_5
#define COL3 GPIO_Pin_6
#define COL4 GPIO_Pin_7
GPIO_InitTypeDef GPIO_InitStructure;
void delay_ms(uint32_t ms)
{
uint32_t i, j;
for (i = 0; i < ms; i++)
for (j = 0; j < 7200; j++);
}
void init_gpio(void)
{
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Pin = ROW1 | ROW2 | ROW3 | ROW4;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_InitStructure.GPIO_Pin = COL1 | COL2 | COL3 | COL4;
GPIO_Init(GPIOA, &GPIO_InitStructure);
}
int main(void)
{
init_gpio();
uint8_t keymap[4][4] = {
{1, 2, 3, 0x0a},
{4, 5, 6, 0x0b},
{7, 8, 9, 0x0c},
{0x0e, 0, 0x0f, 0x0d}
};
while (1)
{
uint8_t row, col, key;
for (row = 0; row < 4; row++)
{
GPIO_ResetBits(GPIOA, ROW1 << row);
delay_ms(1);
if (GPIO_ReadInputDataBit(GPIOA, COL1))
col = 0;
else if (GPIO_ReadInputDataBit(GPIOA, COL2))
col = 1;
else if (GPIO_ReadInputDataBit(GPIOA, COL3))
col = 2;
else if (GPIO_ReadInputDataBit(GPIOA, COL4))
col = 3;
else
continue;
key = keymap[row][col];
// TODO: do something with the key
delay_ms(50);
}
}
}
```
相关推荐
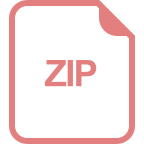
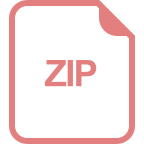
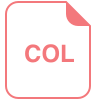
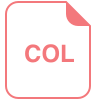
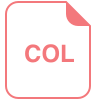
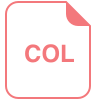
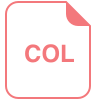









