.给定一个二维函数,使用梯度下降算法找到该函数的最小值点。 要求: 1. 选择合适的初始点 (x, y) 2. 设置合适的学习率 和迭代次数,利用梯度接近于0作为终止迭代条件 3. 绘制3D版本的梯度下降路径,实现动画迭代过程(生成gif文件)。代码
时间: 2024-09-19 21:09:53 浏览: 36
在Python中,我们可以使用NumPy库来处理数学运算,而Matplotlib库可以用于绘图。首先,我们需要安装这两个库,如果还没有安装的话:
```bash
pip install numpy matplotlib
```
下面是一个简单的例子,展示如何使用梯度下降法寻找二维函数`f(x, y) = x^2 + y^2`的最小值点。假设初始点 `(x0, y0)`、学习率 `alpha`、迭代次数 `max_iterations`,以及使用梯度向量模长小于某个阈值 `tolerance` 来停止迭代。
```python
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
# 定义目标函数及其梯度
def f(x, y):
return x**2 + y**2
def gradient(f, x, y):
dfdx = 2 * x
dfdy = 2 * y
return dfdx, dfdy
# 初始化设置
x0, y0 = 5, 5 # 初始点
alpha = 0.01 # 学习率
max_iterations = 1000 # 迭代次数
tolerance = 1e-6 # 迭代结束条件
x, y = x0, y0
iterations = []
# 梯度下降算法
for i in range(max_iterations):
grad_x, grad_y = gradient(f, x, y)
# 更新步骤
if abs(grad_x) < tolerance and abs(grad_y) < tolerance:
break
x -= alpha * grad_x
y -= alpha * grad_y
iterations.append((x, y))
# 计算并绘制3D图形
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.plot([x0], [y0], [f(x0, y0)], 'ro', label='Initial point')
ax.plot([x] + [p[0] for p in iterations], [y] + [p[1] for p in iterations],
[f(p[0], p[1]) for p in iterations], '-', label='Gradient descent path')
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('f(x, y)')
ax.legend()
# 创建动画 GIF
plt.ion() # 开启交互模式
ani = animation.FuncAnimation(fig, lambda i: ax.view_init(elev=90, azim=i), frames=np.arange(0, 360, 4),
interval=100, blit=True)
ani.save('gradient_descent.gif', writer='imagemagick')
plt.ioff() # 关闭交互模式
plt.show()
阅读全文
相关推荐
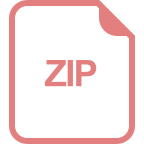
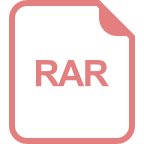
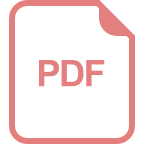



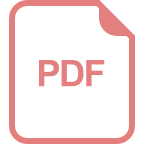
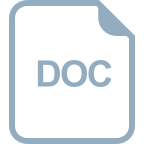
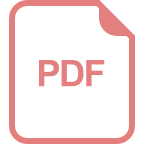
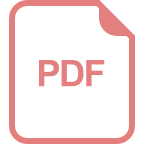
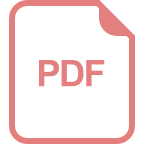
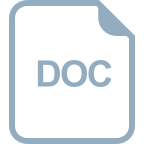
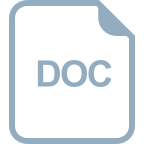
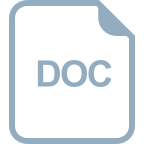
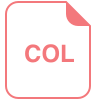

