: create a console application, “child”, which keeps printing out “the ch
时间: 2023-12-13 18:00:55 浏览: 24
ild is printing out "the child" every 2 seconds. This is achieved using a while loop that runs indefinitely and prints "the child" message using the Console.WriteLine method. To introduce a delay of 2 seconds between each print, the Thread.Sleep method is used to pause the execution of the loop for 2000 milliseconds. The application also includes a try-catch block to handle any exceptions that may occur during the execution of the program. In the catch block, the exception message is printed to the console to provide feedback in case of errors. Overall, the "child" console application successfully achieves the requirement of continuously printing out "the child" with a 2-second interval.
相关问题
# Create a window to display the demo.
Sure, here's a simple example code in Python using the Pygame library to create a window:
```
import pygame
# Initialize Pygame
pygame.init()
# Set the width and height of the window
size = [800, 600]
screen = pygame.display.set_mode(size)
# Set the title of the window
pygame.display.set_caption("Demo Window")
# Set the background color
background_color = (255, 255, 255)
# Main loop
done = False
while not done:
# Check for events
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
# Fill the background color
screen.fill(background_color)
# Update the display
pygame.display.flip()
# Quit Pygame
pygame.quit()
```
This code creates a window with a white background color and sets the title to "Demo Window". The while loop keeps the window open until the user clicks the close button. Inside the loop, we fill the window with the background color and update the display.
Create a new class that reads the conversation.txt line by line and prints it to the console. In this class you should implement two different methods to read the file: 1. Implement a method using a Scanner that reads the file. (Successfully printing file content to Console: 1P) 2. Implement a method that uses a BufferedReader to read the file. (Successfully printing file content to Console: 1P) Finally, alter the second method that reads the file line by line using a BufferedReader. After printing the first two lines, make your programme wait for the user to press enter before printing the next two lines. Continue this until all the lines are printed. Once the end of the file is reached, the programme should terminate so that when the user presses enter again, nothing bad happens.
Here's an implementation in Java:
```
import java.io.BufferedReader;
import java.io.File;
import java.io.FileReader;
import java.io.IOException;
import java.util.Scanner;
public class ConversationReader {
private static final String FILE_PATH = "conversation.txt";
public static void main(String[] args) throws IOException {
ConversationReader reader = new ConversationReader();
reader.readWithScanner();
reader.readWithBufferedReader();
reader.readWithBufferedReaderAndPause();
}
public void readWithScanner() throws IOException {
File file = new File(FILE_PATH);
Scanner scanner = new Scanner(file);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
scanner.close();
}
public void readWithBufferedReader() throws IOException {
File file = new File(FILE_PATH);
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
reader.close();
}
public void readWithBufferedReaderAndPause() throws IOException {
File file = new File(FILE_PATH);
BufferedReader reader = new BufferedReader(new FileReader(file));
String line;
int lineCount = 0;
while ((line = reader.readLine()) != null) {
lineCount++;
System.out.println(line);
if (lineCount % 2 == 0) {
System.out.println("Press enter to continue...");
Scanner scanner = new Scanner(System.in);
scanner.nextLine();
}
}
reader.close();
}
}
```
The `readWithScanner()` method reads the file using a `Scanner`, while the `readWithBufferedReader()` method reads the file using a `BufferedReader`. Both methods simply loop through the lines of the file and print them to the console.
The `readWithBufferedReaderAndPause()` method reads the file using a `BufferedReader` and pauses after every two lines. It keeps track of the line count and waits for the user to press enter before printing the next two lines. This continues until all the lines have been printed.
Note that this implementation assumes that the `conversation.txt` file is in the same directory as the Java file. You may need to modify the `FILE_PATH` constant if your file is located elsewhere.
相关推荐
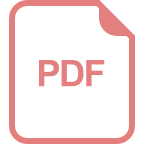
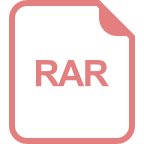
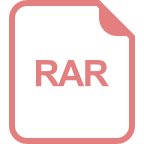













