Suppose that our banking system requires the ability to make joint accounts. Define a function make_joint that takes three arguments. A password-protected withdraw function, The password with which that withdraw function was defined, and A new password that can also access the original account. If the password is incorrect or cannot be verified because the underlying account is locked, the make_joint should propagate the error. Otherwise, it returns a withdraw function that provides additional access to the original account using either the new or old password. Both functions draw from the same balance. Incorrect passwords provided to either function will be stored and cause the functions to be locked after three wrong attempts.
时间: 2024-02-24 07:00:07 浏览: 189
Here is one possible implementation of the `make_joint` function in Python:
```python
def make_joint(withdraw_fn, password, new_password):
# Define a counter to keep track of the number of incorrect password attempts
incorrect_passwords = [0]
# Define a helper function to check the password and call the original withdraw function
def withdraw(amount, entered_password):
if entered_password not in [password, new_password]:
incorrect_passwords[0] += 1
if incorrect_passwords[0] >= 3:
withdraw_fn('LOCKED')
return 'INCORRECT PASSWORD'
else:
return withdraw_fn(amount, password)
# Call the original withdraw function with the correct password to ensure it is valid
if withdraw(0, password) == 'INCORRECT PASSWORD':
return 'INVALID PASSWORD'
# Return the new withdraw function that can be used with either password
return withdraw
```
The `make_joint` function takes in a withdraw function, a password, and a new password, and it returns a new withdraw function that can be used with either password. The function keeps track of the number of incorrect password attempts using a list with one value, `incorrect_passwords`, which is initialized to `[0]`. If the incorrect password is entered three times, the account is locked by calling the original withdraw function with the `'LOCKED'` argument.
The `withdraw` helper function checks the entered password against the original password and the new password. If the entered password is incorrect, it increments the `incorrect_passwords` counter and returns `'INCORRECT PASSWORD'`. If the counter reaches 3, the function locks the account by calling the original withdraw function with the `'LOCKED'` argument. Otherwise, the function calls the original withdraw function with the requested amount and the original password.
Finally, the function calls the original withdraw function with 0 and the original password to ensure that the provided password is valid. If it is not, the function returns `'INVALID PASSWORD'`. Otherwise, it returns the `withdraw` helper function that can be used with either password.
阅读全文
相关推荐
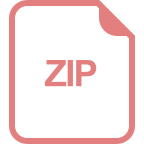
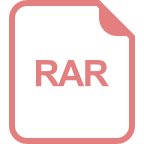









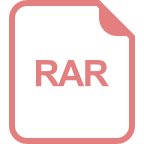
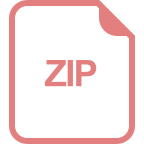
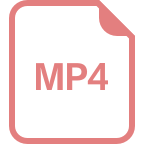