gdal找到dem中距离待求点最近的已知高程点c++
时间: 2024-05-16 09:15:24 浏览: 5
可以使用GDAL库中的GDALRasterBand类来实现这个功能。以下是一个简单的示例代码:
```c++
#include <iostream>
#include "gdal_priv.h"
#include "cpl_conv.h" // for CPLMalloc()
int main()
{
// Open the DEM file
GDALAllRegister();
GDALDataset *pDataset = (GDALDataset *) GDALOpen("dem.tif", GA_ReadOnly);
if (pDataset == NULL)
{
std::cerr << "Failed to open DEM file!" << std::endl;
return 1;
}
// Get the raster band
GDALRasterBand *pBand = pDataset->GetRasterBand(1);
// Get the geo-transform
double adfGeoTransform[6];
pDataset->GetGeoTransform(adfGeoTransform);
// Define the point to query
double x = 123.45;
double y = 67.89;
// Convert the point to pixel coordinates
int nPixel, nLine;
pBand->GetGeoTransform(adfGeoTransform, x, y, &nPixel, &nLine);
// Read the pixel value
float fValue;
pBand->RasterIO(GF_Read, nPixel, nLine, 1, 1, &fValue, 1, 1, GDT_Float32, 0, 0);
// Print the pixel value
std::cout << "Pixel value at (" << x << ", " << y << ") is " << fValue << std::endl;
// Close the dataset
GDALClose(pDataset);
return 0;
}
```
该示例代码首先打开了DEM文件,然后获取了第一个波段(假设DEM只有一个波段)。接下来,它获取了DEM的地理变换,用于将点坐标转换为像素坐标。然后,它读取了像素值,并将其输出到控制台。
如果要找到离查询点最近的已知高程点,您可以在DEM中查询一系列点,并找到其中距离查询点最近的点。以下是一个示例代码:
```c++
#include <iostream>
#include "gdal_priv.h"
#include "cpl_conv.h" // for CPLMalloc()
#include <vector>
#include <cmath>
struct Point
{
double x;
double y;
float z;
};
int main()
{
// Open the DEM file
GDALAllRegister();
GDALDataset *pDataset = (GDALDataset *) GDALOpen("dem.tif", GA_ReadOnly);
if (pDataset == NULL)
{
std::cerr << "Failed to open DEM file!" << std::endl;
return 1;
}
// Get the raster band
GDALRasterBand *pBand = pDataset->GetRasterBand(1);
// Get the geo-transform
double adfGeoTransform[6];
pDataset->GetGeoTransform(adfGeoTransform);
// Define the query point
double x = 123.45;
double y = 67.89;
// Convert the query point to pixel coordinates
int nPixel, nLine;
pBand->GetGeoTransform(adfGeoTransform, x, y, &nPixel, &nLine);
// Define the search radius in pixels
int nRadius = 10;
// Define the search area in pixels
int nLeft = nPixel - nRadius;
int nTop = nLine - nRadius;
int nWidth = 2 * nRadius + 1;
int nHeight = 2 * nRadius + 1;
// Read the pixel values in the search area
std::vector<float> vecValues(nWidth * nHeight);
pBand->RasterIO(GF_Read, nLeft, nTop, nWidth, nHeight, &vecValues[0], nWidth, nHeight, GDT_Float32, 0, 0);
// Convert the search area to geographic coordinates
std::vector<Point> vecPoints(nWidth * nHeight);
for (int i = 0; i < nWidth; ++i)
{
for (int j = 0; j < nHeight; ++j)
{
int nIndex = i * nHeight + j;
double x = adfGeoTransform[0] + (nLeft + i) * adfGeoTransform[1] + (nTop + j) * adfGeoTransform[2];
double y = adfGeoTransform[3] + (nLeft + i) * adfGeoTransform[4] + (nTop + j) * adfGeoTransform[5];
vecPoints[nIndex].x = x;
vecPoints[nIndex].y = y;
vecPoints[nIndex].z = vecValues[nIndex];
}
}
// Find the point closest to the query point
Point closestPoint;
closestPoint.z = std::numeric_limits<float>::max();
for (const auto &point : vecPoints)
{
double dx = point.x - x;
double dy = point.y - y;
double distance = std::sqrt(dx*dx + dy*dy);
if (distance < nRadius && point.z < closestPoint.z)
{
closestPoint = point;
}
}
// Print the closest point
std::cout << "Closest point to (" << x << ", " << y << ") is (" << closestPoint.x << ", " << closestPoint.y << ", " << closestPoint.z << ")" << std::endl;
// Close the dataset
GDALClose(pDataset);
return 0;
}
```
该示例代码首先定义了要查询的点,并将其转换为像素坐标。然后,它定义了一个搜索半径,并使用该半径在DEM中查询像素值。接下来,它将搜索区域中的每个像素转换为地理坐标,并将其保存在一个点向量中。然后,它找到最靠近查询点的点,并将其输出到控制台。
请注意,此示例代码仅在搜索区域内搜索点。如果您需要在整个DEM中搜索点,则需要更改搜索区域并读取整个DEM,这可能会非常耗时。
相关推荐
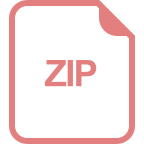














