定义一个名为Point类表示点,构造方法public Point(double x,double y),访问方法有getX(),getY()。(1)覆盖从超类继承的toString()方法,调用该方法返回类名及点的x和y坐标值。(2)覆盖equals()方法,当两个点的x和y坐标分别相等,方法返回true,否则返回false。(3)覆盖hashCode()方法,要求使用Objects类的hash()方法计算哈希码。(4)编写main()方法,测试上述几个方法的使用。
时间: 2024-02-21 13:59:04 浏览: 115
Point类的定义如下:
```java
import java.util.Objects;
public class Point {
private double x;
private double y;
public Point(double x, double y) {
this.x = x;
this.y = y;
}
public double getX() {
return x;
}
public double getY() {
return y;
}
@Override
public String toString() {
return "Point(" + x + ", " + y + ")";
}
@Override
public boolean equals(Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof Point)) {
return false;
}
Point other = (Point) obj;
return Double.compare(other.x, x) == 0 && Double.compare(other.y, y) == 0;
}
@Override
public int hashCode() {
return Objects.hash(x, y);
}
public static void main(String[] args) {
Point p1 = new Point(1.0, 2.0);
Point p2 = new Point(1.0, 2.0);
Point p3 = new Point(2.0, 3.0);
System.out.println(p1.equals(p2)); // true
System.out.println(p1.equals(p3)); // false
System.out.println(p1.hashCode() == p2.hashCode()); // true
System.out.println(p1.hashCode() == p3.hashCode()); // false
System.out.println(p1.toString()); // Point(1.0, 2.0)
}
}
```
上述代码中,`Point` 类有两个私有属性 `x` 和 `y` 分别表示点的 x 坐标和 y 坐标。`Point` 类有一个构造方法 `public Point(double x, double y)` 用于创建具有指定坐标的点对象。`Point` 类还有两个访问方法 `public double getX()` 和 `public double getY()` 分别用于获取点的 x 坐标和 y 坐标。
`Point` 类覆盖了从超类继承的 `toString()` 方法,调用该方法返回类名及点的 x 和 y 坐标值。`Point` 类还覆盖了 `equals()` 方法,当两个点的 x 和 y 坐标分别相等时,方法返回 `true`,否则返回 `false`。`Point` 类覆盖了 `hashCode()` 方法,使用 `Objects` 类的 `hash()` 方法计算哈希码。
在 `main()` 方法中测试了 `equals()` 方法、`hashCode()` 方法以及 `toString()` 方法的使用。
相关推荐
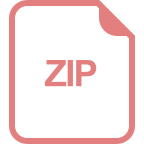
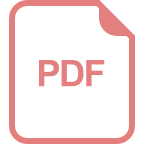
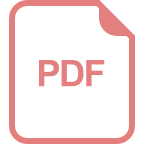














