用C++写一个链式队列
时间: 2023-12-27 13:43:55 浏览: 76
好的,以下是用C++实现的链式队列代码:
```c++
#include <iostream>
using namespace std;
// 定义链表节点结构体
struct Node {
int data;
Node* next;
};
// 定义队列结构体
struct Queue {
Node* front;
Node* rear;
};
// 初始化队列
void initQueue(Queue& q) {
q.front = q.rear = NULL;
}
// 判断队列是否为空
bool isEmpty(Queue q) {
return q.front == NULL;
}
// 入队
void enQueue(Queue& q, int x) {
Node* newNode = new Node;
newNode->data = x;
newNode->next = NULL;
if (isEmpty(q)) {
q.front = q.rear = newNode;
} else {
q.rear->next = newNode;
q.rear = newNode;
}
}
// 出队
void deQueue(Queue& q) {
if (isEmpty(q)) {
cout << "队列为空,无法出队!" << endl;
} else {
Node* p = q.front;
q.front = q.front->next;
if (q.front == NULL) {
q.rear = NULL;
}
delete p;
}
}
// 获取队头元素
int front(Queue q) {
if (isEmpty(q)) {
cout << "队列为空!" << endl;
return -1;
} else {
return q.front->data;
}
}
// 获取队列长度
int size(Queue q) {
int len = 0;
Node* p = q.front;
while (p != NULL) {
len++;
p = p->next;
}
return len;
}
// 输出队列
void printQueue(Queue q) {
if (isEmpty(q)) {
cout << "队列为空!" << endl;
} else {
Node* p = q.front;
while (p != NULL) {
cout << p->data << " ";
p = p->next;
}
cout << endl;
}
}
int main() {
Queue q;
initQueue(q);
enQueue(q, 1);
enQueue(q, 2);
enQueue(q, 3);
cout << "队列长度为:" << size(q) << endl;
cout << "队头元素为:" << front(q) << endl;
printQueue(q);
deQueue(q);
cout << "出队后队头元素为:" << front(q) << endl;
printQueue(q);
return 0;
}
```
以上代码实现了一个基本的链式队列,包含了初始化队列、判断队列是否为空、入队、出队、获取队头元素、获取队列长度和输出队列等功能。
阅读全文
相关推荐
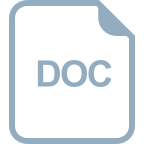
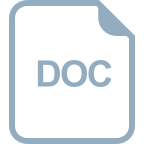
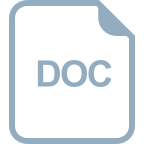


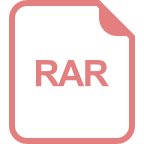
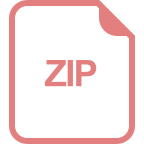
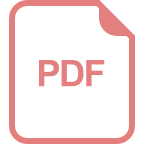
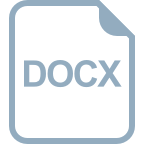
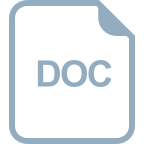
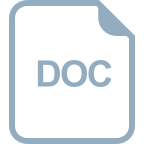






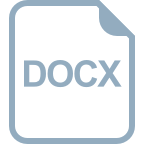