cshap查询时间一个时间段是否再数据库内有重复,如果有重复,重复时间修改延后至查询时间结束后,如果修改时间与数据库内有重复再次修改
时间: 2023-05-28 22:02:10 浏览: 132
可以通过以下步骤实现:
1. 编写查询语句,查询数据库内与待修改时间段有重叠的记录。可以使用 SQL 语句中的 BETWEEN 运算符或相应的日期函数来完成。
2. 如果查询结果不为空,则表示有重叠的记录,需要进行延后处理。可以将待修改的时间段延后至查询时间段的结束时间。
3. 再次查询数据库,判断延后后的时间段是否与现有记录重叠。
4. 如果仍然有重叠,重复步骤2和3,直到没有重叠为止。
5. 如果没有重叠,可以将数据库记录更新为延后后的时间段。
具体实现可以参考以下代码(假设数据库中记录时间段表示为 start_time 和 end_time):
```
DateTime startTime = ...; // 待修改的时间段起始时间
DateTime endTime = ...; // 待修改的时间段结束时间
// 查询与待修改时间段重叠的记录
string query = "SELECT * FROM table_name WHERE start_time <= @end_time AND end_time >= @start_time";
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@start_time", startTime);
command.Parameters.AddWithValue("@end_time", endTime);
SqlDataReader reader = command.ExecuteReader();
if (reader.HasRows)
{
// 有重叠记录,需要延后待修改时间段
while (reader.Read())
{
DateTime existingStartTime = reader.GetDateTime(0);
DateTime existingEndTime = reader.GetDateTime(1);
if (existingEndTime >= startTime)
{
startTime = existingEndTime.AddMinutes(1); // 加1分钟作为新的起始时间
}
if (endTime <= existingEndTime)
{
endTime = existingEndTime.AddMinutes(1); // 加1分钟作为新的结束时间
}
}
reader.Close();
// 再次查询是否有重叠记录
bool overlapped = true;
while (overlapped)
{
query = "SELECT COUNT(*) FROM table_name WHERE start_time <= @end_time AND end_time >= @start_time";
command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@start_time", startTime);
command.Parameters.AddWithValue("@end_time", endTime);
int count = (int)command.ExecuteScalar();
if (count == 0)
{
// 没有重叠记录,更新数据库记录
query = "UPDATE table_name SET start_time = @new_start_time, end_time = @new_end_time WHERE start_time = @old_start_time AND end_time = @old_end_time";
command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@new_start_time", startTime);
command.Parameters.AddWithValue("@new_end_time", endTime);
command.Parameters.AddWithValue("@old_start_time", reader.GetDateTime(0));
command.Parameters.AddWithValue("@old_end_time", reader.GetDateTime(1));
int rowsAffected = command.ExecuteNonQuery();
if (rowsAffected > 0)
{
overlapped = false;
}
}
else
{
// 有重叠记录,继续延后待修改时间段
startTime = endTime.AddMinutes(1);
endTime = endTime.AddMinutes(1);
}
}
}
else
{
// 没有重叠记录,直接更新数据库记录
query = "UPDATE table_name SET start_time = @new_start_time, end_time = @new_end_time WHERE start_time = @old_start_time AND end_time = @old_end_time";
command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@new_start_time", startTime);
command.Parameters.AddWithValue("@new_end_time", endTime);
command.Parameters.AddWithValue("@old_start_time", reader.GetDateTime(0));
command.Parameters.AddWithValue("@old_end_time", reader.GetDateTime(1));
int rowsAffected = command.ExecuteNonQuery();
}
```
阅读全文
相关推荐
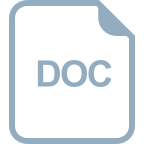
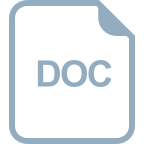
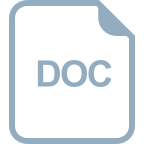














