cshap 再数据库中插入一段时间,如果有重复则将查询到的时间顺延插入的天数,顺延后的时间如果和数据库内的其他时间有重复则将其他时间顺延 以此类推
时间: 2023-05-26 09:04:39 浏览: 90
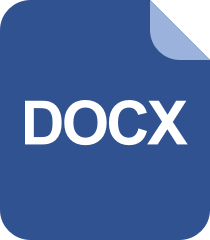
SOAP教程C++CSHAP
以下是一个示例代码,实现在数据库中插入一段时间并进行顺延:
```csharp
using System;
using System.Data.SqlClient;
namespace ConsoleApp
{
class Program
{
static void Main(string[] args)
{
// 假设要插入的时间段为从 2022 年 1 月 1 日到 2022 年 1 月 5 日
DateTime startDate = new DateTime(2022, 1, 1);
DateTime endDate = new DateTime(2022, 1, 5);
// 假设要插入的数据表名为 time_table,日期字段名为 date
string tableName = "time_table";
string dateColumn = "date";
// 假设要顺延的天数为 2 天
int delayDays = 2;
// 创建数据库连接
SqlConnection connection = new SqlConnection("your_connection_string");
connection.Open();
// 查询数据库中已有的日期并进行顺延
DateTime currentDt = startDate;
while (currentDt <= endDate)
{
SqlCommand command = new SqlCommand($"SELECT COUNT(*) FROM {tableName} WHERE {dateColumn} = @date", connection);
command.Parameters.AddWithValue("@date", currentDt);
int count = (int)command.ExecuteScalar();
if (count > 0)
{
// 如果查询到的日期已存在,则将日期顺延 delayDays 天
currentDt = currentDt.AddDays(delayDays);
}
else
{
// 如果查询到的日期不存在,则将当前日期插入数据库中,并将 currentDt 设为下一天的日期
command = new SqlCommand($"INSERT INTO {tableName} ({dateColumn}) VALUES (@date)", connection);
command.Parameters.AddWithValue("@date", currentDt);
command.ExecuteNonQuery();
currentDt = currentDt.AddDays(1);
}
}
connection.Close();
Console.WriteLine("Done");
}
}
}
```
以上代码中,首先取得要插入的时间段的开始时间和结束时间,还有数据表名和日期字段名以及要顺延的天数。然后用一个循环遍历要插入的时间段中的每一天,每次循环先查询该日期在数据库中是否已经存在,如果存在则将日期顺延 delayDays 天,继续查询。如果不存在,则将该日期插入数据库中,并将当前日期设为下一天的日期。在查询和插入数据库的时候要使用参数化 SQL,防止 SQL 注入攻击。
阅读全文
相关推荐
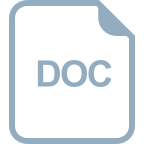
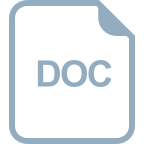















