cshap list判断重复数据
时间: 2023-08-20 14:27:11 浏览: 38
在 C# 中可以使用 LINQ 来判断列表中是否存在重复的数据。具体来说,可以使用 `GroupBy` 方法将列表按照元素进行分组,然后判断分组后的元素数量是否大于 1,如果大于 1,就说明该元素存在重复。下面是一个示例代码:
```
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main(string[] args)
{
List<int> myList = new List<int> { 1, 2, 3, 3, 4, 4, 5 };
var duplicates = myList.GroupBy(x => x)
.Where(g => g.Count() > 1)
.Select(g => g.Key);
foreach (var duplicate in duplicates)
{
Console.WriteLine("Duplicate element: {0}", duplicate);
}
}
}
```
输出结果为:
```
Duplicate element: 3
Duplicate element: 4
```
如果只需要判断列表中是否存在重复元素,可以使用 `Any` 方法来判断是否存在分组后元素数量大于 1 的情况,下面是一个示例代码:
```
using System;
using System.Collections.Generic;
using System.Linq;
class Program
{
static void Main(string[] args)
{
List<int> myList = new List<int> { 1, 2, 3, 3, 4, 4, 5 };
bool hasDuplicates = myList.GroupBy(x => x)
.Any(g => g.Count() > 1);
Console.WriteLine("Has duplicates: {0}", hasDuplicates);
}
}
```
输出结果为:
```
Has duplicates: True
```
相关推荐
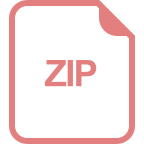














