openssl中通过ECDH算法对方公钥和己方公钥计算共享密钥的函数
时间: 2024-05-21 14:17:36 浏览: 285
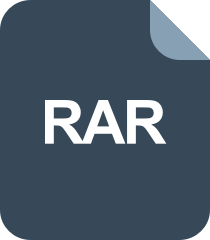
DH.rar_DH_DH加密_dh rsa_dh共享key编码_共享密钥

在 OpenSSL 中,可以使用以下函数通过 ECDH 算法计算共享密钥:
1. `EC_KEY_compute_key()`:该函数可以计算 ECDH 共享密钥,并将结果存储在指定的缓冲区中。该函数的参数包括:
- `out`:指向输出缓冲区的指针。
- `outlen`:输出缓冲区的长度。
- `pub_key`:对方公钥的 `EC_POINT` 对象。
- `ecdh`:己方的 `EC_KEY` 对象。
- `kdf`:指定密钥派生函数,可以为 `NULL`。
2. `ECDH_compute_key()`:该函数也可以计算 ECDH 共享密钥,并将结果存储在指定的缓冲区中。该函数的参数包括:
- `out`:指向输出缓冲区的指针。
- `outlen`:输出缓冲区的长度。
- `pub_key`:对方公钥的 `EC_POINT` 对象。
- `ecdh`:己方的 `EC_KEY` 对象。
- `kdf`:指定密钥派生函数,可以为 `NULL`。
这两个函数的主要区别在于 `EC_KEY_compute_key()` 函数需要指定己方的 `EC_KEY` 对象,而 `ECDH_compute_key()` 函数需要通过一个 `EVP_PKEY` 对象来传递己方的公钥。另外,`ECDH_compute_key()` 函数还可以支持其他类型的密钥交换算法,例如 DH 算法。
阅读全文
相关推荐
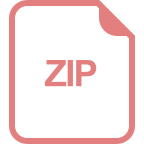
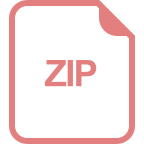
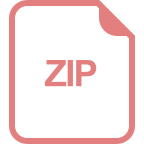
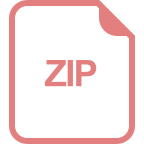
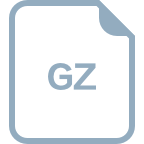
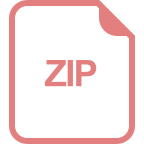
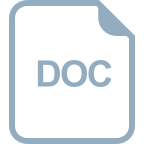
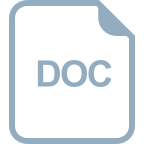
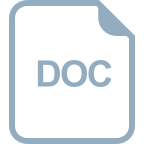
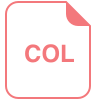





