多变量公钥加密算法C++实现
时间: 2023-08-05 15:05:55 浏览: 71
以下是一个基于椭圆曲线的多变量公钥加密算法(ECMQV)的 C++ 实现:
```c++
#include <iostream>
#include <string>
#include <utility>
#include <vector>
#include <openssl/ec.h>
#include <openssl/ecdh.h>
#include <openssl/evp.h>
#include <openssl/rand.h>
using namespace std;
// 椭圆曲线参数
const char* curve_name = "secp256k1";
// 生成椭圆曲线密钥对
pair<EC_KEY*, EC_POINT*> generate_keypair() {
// 创建椭圆曲线参数
EC_GROUP* group = EC_GROUP_new_by_curve_name(NID_secp256k1);
// 生成私钥
EC_KEY* key = EC_KEY_new();
EC_KEY_set_group(key, group);
EC_KEY_generate_key(key);
// 生成公钥
EC_POINT* pub_key = EC_POINT_new(group);
EC_POINT_copy(pub_key, EC_KEY_get0_public_key(key));
return make_pair(key, pub_key);
}
// 计算共享密钥
vector<unsigned char> compute_shared_secret(EC_KEY* my_key, EC_POINT* my_pub_key, EC_KEY* other_key, EC_POINT* other_pub_key) {
// 创建椭圆曲线参数
EC_GROUP* group = EC_GROUP_new_by_curve_name(NID_secp256k1);
// 计算共享密钥
size_t len = ECDH_compute_key(NULL, 0, other_pub_key, my_key, NULL);
vector<unsigned char> shared_secret(len);
ECDH_compute_key(shared_secret.data(), shared_secret.size(), other_pub_key, my_key, NULL);
return shared_secret;
}
// 计算哈希值
vector<unsigned char> hash_message(string message) {
EVP_MD_CTX* mdctx = EVP_MD_CTX_new();
EVP_DigestInit(mdctx, EVP_sha256());
EVP_DigestUpdate(mdctx, message.c_str(), message.length());
vector<unsigned char> hash(EVP_MD_size(EVP_sha256()));
unsigned int hash_len;
EVP_DigestFinal(mdctx, hash.data(), &hash_len);
EVP_MD_CTX_free(mdctx);
return hash;
}
int main() {
// 生成 Alice 和 Bob 的密钥对
auto alice_keypair = generate_keypair();
auto bob_keypair = generate_keypair();
// Alice 和 Bob 互相交换公钥,计算共享密钥
auto alice_shared_secret = compute_shared_secret(alice_keypair.first, alice_keypair.second, bob_keypair.first, bob_keypair.second);
auto bob_shared_secret = compute_shared_secret(bob_keypair.first, bob_keypair.second, alice_keypair.first, alice_keypair.second);
// 比较 Alice 和 Bob 计算出的共享密钥是否相同
if (alice_shared_secret == bob_shared_secret) {
cout << "Shared secret: ";
for (auto b : alice_shared_secret) {
printf("%02x", b);
}
cout << endl;
} else {
cout << "Error: shared secrets do not match" << endl;
}
// 使用共享密钥加密和解密消息
string message = "Hello World!";
vector<unsigned char> shared_secret = alice_shared_secret; // 使用 Alice 和 Bob 之间计算出的共享密钥
vector<unsigned char> iv(16); // 初始化向量
RAND_bytes(iv.data(), iv.size()); // 生成随机的初始化向量
vector<unsigned char> hash = hash_message(message); // 计算消息的哈希值
vector<unsigned char> key(hash.begin(), hash.begin() + 16); // 从哈希值中提取加密密钥
vector<unsigned char> ciphertext; // 加密后的消息
EVP_CIPHER_CTX* ctx = EVP_CIPHER_CTX_new();
EVP_EncryptInit_ex(ctx, EVP_aes_128_cbc(), NULL, key.data(), iv.data());
int out_len;
ciphertext.resize(message.length() + EVP_CIPHER_CTX_block_size(ctx));
EVP_EncryptUpdate(ctx, ciphertext.data(), &out_len, (const unsigned char*)message.c_str(), message.length());
int ciphertext_len = out_len;
EVP_EncryptFinal_ex(ctx, ciphertext.data() + ciphertext_len, &out_len);
ciphertext_len += out_len;
ciphertext.resize(ciphertext_len);
EVP_CIPHER_CTX_free(ctx);
cout << "Ciphertext: ";
for (auto b : ciphertext) {
printf("%02x", b);
}
cout << endl;
string decrypted_message; // 解密后的消息
ctx = EVP_CIPHER_CTX_new();
EVP_DecryptInit_ex(ctx, EVP_aes_128_cbc(), NULL, key.data(), iv.data());
decrypted_message.resize(ciphertext.size());
EVP_DecryptUpdate(ctx, (unsigned char*)decrypted_message.c_str(), &out_len, ciphertext.data(), ciphertext_len);
int decrypted_len = out_len;
EVP_DecryptFinal_ex(ctx, (unsigned char*)decrypted_message.c_str() + decrypted_len, &out_len);
decrypted_len += out_len;
decrypted_message.resize(decrypted_len);
EVP_CIPHER_CTX_free(ctx);
cout << "Decrypted message: " << decrypted_message << endl;
return 0;
}
```
在这个实现中,我们使用 OpenSSL 库中的 ECDSA 和 ECDH 函数来进行椭圆曲线加密和密钥交换。我们首先生成 Alice 和 Bob 的密钥对,然后它们互相交换公钥,并计算出共享密钥。接着,我们使用共享密钥加密和解密消息。在这个实现中,我们使用 AES-128-CBC 模式来加密消息,并使用 SHA-256 哈希算法计算消息的哈希值,从中提取加密密钥。需要注意的是,我们需要生成一个随机的初始化向量,以增加加密的安全性。
相关推荐
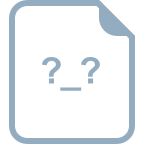
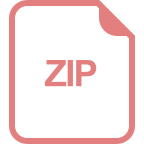
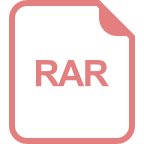














