ElGamal公钥加密算法 C++实现
时间: 2023-07-11 12:33:13 浏览: 81
下面给出一个使用 Crypto++ 库实现 ElGamal 公钥加密算法的示例代码,供参考:
```cpp
#include <iostream>
#include <cryptopp/elgamal.h>
#include <cryptopp/osrng.h>
using namespace CryptoPP;
using namespace std;
int main()
{
// 定义 ElGamal 密钥
AutoSeededRandomPool rng; // 随机数生成器
ElGamalKeys::PrivateKey privateKey;
ElGamalKeys::PublicKey publicKey;
privateKey.GenerateRandomWithKeySize(rng, 256);
publicKey.AssignFrom(privateKey);
// 输出公钥和私钥
cout << "Private key: " << endl;
cout << "x: " << privateKey.GetPrivateExponent() << endl;
cout << "p: " << privateKey.GetGroupParameters().GetModulus() << endl;
cout << "Public key: " << endl;
cout << "y: " << publicKey.GetPublicElement() << endl;
cout << "p: " << publicKey.GetGroupParameters().GetModulus() << endl;
// 加密和解密
string message = "Hello, world!";
ElGamal::Encryptor encryptor(publicKey);
ElGamal::Decryptor decryptor(privateKey);
SecByteBlock ciphertext(encryptor.CiphertextLength(message.size()));
encryptor.Encrypt(rng, (const byte*)message.data(), message.size(), ciphertext);
SecByteBlock plaintext(encryptor.MaxPlaintextLength(ciphertext.size()));
size_t decryptedSize = decryptor.Decrypt(rng, ciphertext, ciphertext.size(), plaintext);
plaintext.resize(decryptedSize);
// 输出加密结果和解密结果
cout << "Message: " << message << endl;
cout << "Ciphertext: " << (const char*)ciphertext.data() << endl;
cout << "Plaintext: " << (const char*)plaintext.data() << endl;
return 0;
}
```
上述代码实现了使用 256 位素数进行 ElGamal 公钥加密的功能。其中,AutoSeededRandomPool 类用于生成随机数,ElGamalKeys::PrivateKey 和 ElGamalKeys::PublicKey 分别表示私钥和公钥,ElGamal::Encryptor 和 ElGamal::Decryptor 分别用于加密和解密。具体使用方法可以参考 Crypto++ 的官方文档。
相关推荐
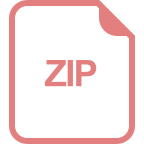
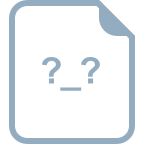














