‘std::any’ is only available from C++17 onwards,我应该怎么修改CMAKEList
时间: 2023-09-19 17:06:13 浏览: 148
### 回答1:
If you are using a CMake version prior to 3.8, you should update your CMake version to at least 3.8.0, which added support for C++17.
Then, in your CMakeLists.txt file, add the following lines:
```cmake
set(CMAKE_CXX_STANDARD 17)
set(CMAKE_CXX_STANDARD_REQUIRED ON)
```
This sets the C++ standard to 17 and enforces that the compiler uses that standard.
Next, you should check if your compiler supports C++17 features. If you are using GCC or Clang, you should use version 7 or higher. For Visual Studio, you should use version 2019 or higher.
If your compiler does not support C++17, you may need to upgrade your compiler or switch to a different compiler that does support it.
Finally, if you are using any C++17 features that are not supported by older compilers, you should add appropriate feature checks to your code. One way to do this is to use the `__has_cpp_attribute` macro, which checks if a given attribute is supported by the compiler. For example:
```cpp
#if __has_cpp_attribute(maybe_unused)
#define UNUSED [[maybe_unused]]
#else
#define UNUSED
#endif
int main()
{
UNUSED int x = 42;
// ...
}
```
This code defines a macro `UNUSED` that expands to `[[maybe_unused]]` if the `maybe_unused` attribute is supported by the compiler, and expands to nothing otherwise. The `UNUSED` macro can be used to mark variables or functions as unused to suppress compiler warnings.
### 回答2:
若要使用C++17提供的`std::any`功能,需要在CMakeList文件中进行适当的修改。以下是一种可能的修改方式:
1. 首先,在`CMakeLists.txt`文件中添加以下代码,以启用C++17标准:
```
set(CMAKE_CXX_STANDARD 17)
set(CMAKE_CXX_STANDARD_REQUIRED ON)
```
2. 确保CMake版本支持C++17标准。可以使用以下代码检查CMake版本:
```
cmake_minimum_required(VERSION 3.10)
```
3. 如果使用的是旧版本的CMake,需要使用以下代码手动启用C++17支持:
```
if(CMAKE_VERSION VERSION_LESS "3.8.2")
add_compile_options(-std=c++17)
else()
set(CMAKE_CXX_STANDARD 17)
endif()
```
4. 如果项目中有多个目标,需要对每个目标进行修改,以指定使用C++17标准。可以使用以下代码来修改每个目标:
```
set_target_properties(target_name PROPERTIES
CXX_STANDARD 17
CXX_STANDARD_REQUIRED ON
)
```
5. 最后,在项目源文件中的主要C++源文件中,添加以下头文件以包含`std::any`的声明:
```
#include <any>
```
通过这些修改,在构建过程中,CMake将使用C++17标准,并使`std::any`功能在您的项目中可用。请注意,以上代码仅为示例,可能需要根据项目的实际情况进行调整。
### 回答3:
为了在CMakeList中使用`std::any`,你需要将编译器的标准设置为C++17或更高版本。在CMakeList.txt文件中,你可以通过设置CMAKE_CXX_STANDARD变量来实现。
以下是一个示例CMakeList.txt文件,可以用来支持C++17标准的编译:
```cmake
cmake_minimum_required(VERSION 3.16)
project(YourProjectName)
# 设置编译器标准为C++17
set(CMAKE_CXX_STANDARD 17)
# 添加你的源文件
add_executable(YourExecutableName your_source_files.cpp)
```
通过设置`CMAKE_CXX_STANDARD`为17,CMake将使用C++17编译源文件,使你可以使用`std::any`等新的C++17特性。
确保在CMakeLists.txt文件中的适当位置添加以上代码,并且将`YourProjectName`替换为你的项目名称,将`YourExecutableName`替换为你的可执行文件名称,并将`your_source_files.cpp`替换为你的源文件。
注意:你需要确保你的编译器支持C++17标准。如果你的编译器不支持C++17,你需要更新或更换到支持C++17的编译器。
相关推荐
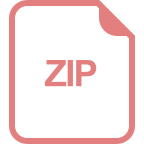
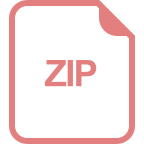
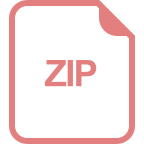








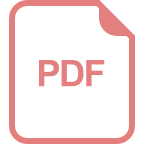
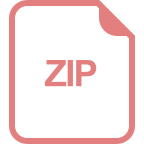
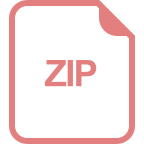
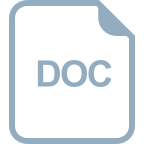
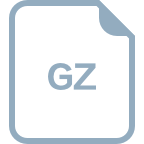
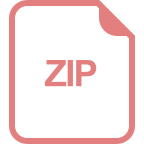