python编写lr算法
时间: 2023-05-23 16:02:34 浏览: 58
可以使用Python编写LR算法。在Python中,可以使用包括Scikit-learn在内的许多机器学习库来实现Logistic Regression模型的快速开发。你需要导入Scikit-learn和一些必要的类(如LogisticRegression和train_test_split)来完成这个任务。然后,使用其它Python库来完成车载工具的特征工程和数据预处理阶段。最后,利用已经准备好的数据在Logistic Regression模型上训练和测试。
相关问题
python lr语法分析实验
Python语言的LR语法分析实验是一项通过Python编程语言实现LR语法分析器的实验。LR语法分析是一种自底向上的语法分析方法,用于分析和验证程序中的语法结构。在实验中,我们可以利用Python语言的灵活性和易用性,编写LR语法分析器的代码,并对其进行测试和验证。
首先,我们需要定义和实现LR分析算法的各个步骤,包括状态转移的规则、LR(0)项集的构建、DFA的生成等。通过Python编程语言的高级特性和丰富的库函数,可以方便地实现这些算法,并且可以通过可视化工具将LR分析器的状态图形象地展现出来,有助于我们更直观地理解算法的执行过程。
其次,我们需要编写语法分析器的输入语法规则和待分析的程序代码。Python语言的简洁和易读性使得编写语法规则变得比较简单,同时也可以使用Python的工具来对待分析的程序代码进行预处理和文法分析,为后续的LR语法分析做准备。
最后,我们可以利用Python语言提供的测试框架对实现的LR语法分析器进行功能和性能测试。通过编写测试用例和使用Python的单元测试框架,可以全面地验证LR语法分析器的正确性和效率,并且可以通过性能分析工具对其性能进行评估和优化。
总的来说,通过Python语言进行LR语法分析实验可以让我们更深入地理解LR语法分析算法的原理和实现,同时也可以充分利用Python语言的优势来简化和优化实验过程,是一项非常有意义的实践活动。
利用随机库make_classification写原生Python编写感知器算法
好的,我来解答您的问题。
感知器算法是一种二分类算法,它的目的是找到一个线性超平面来将两类数据分开。在这里,我将使用 Python 的随机库 make_classification 生成一些随机数据,然后使用原生 Python 编写感知器算法。
首先,我们需要导入必要的库:
```python
import numpy as np
from sklearn.datasets import make_classification
```
然后,我们可以使用 make_classification 生成一些随机数据:
```python
X, y = make_classification(n_samples=100, n_features=2, n_informative=2, n_redundant=0, n_clusters_per_class=1, random_state=42)
```
这会生成 100 个样本,每个样本有 2 个特征,其中 2 个特征是有用的,没有冗余特征,每个类别有 1 个簇,并且使用随机种子 42。
接下来,我们可以定义感知器算法:
```python
class Perceptron:
def __init__(self, learning_rate=0.1, n_iters=1000):
self.lr = learning_rate
self.n_iters = n_iters
self.activation_func = self._unit_step_func
self.weights = None
self.bias = None
def fit(self, X, y):
n_samples, n_features = X.shape
# init parameters
self.weights = np.zeros(n_features)
self.bias = 0
y_ = np.array([1 if i > 0 else 0 for i in y])
# gradient descent
for _ in range(self.n_iters):
for idx, x_i in enumerate(X):
linear_output = np.dot(x_i, self.weights) + self.bias
y_predicted = self.activation_func(linear_output)
update = self.lr * (y_[idx] - y_predicted)
self.weights += update * x_i
self.bias += update
def predict(self, X):
linear_output = np.dot(X, self.weights) + self.bias
y_predicted = self.activation_func(linear_output)
return y_predicted
def _unit_step_func(self, x):
return np.where(x>=0, 1, 0)
```
该算法使用梯度下降法来更新权重和偏置。在初始化时,我们定义了学习率和迭代次数,激活函数使用了阶跃函数,权重和偏置初始化为零。在拟合过程中,我们对每个样本进行迭代,根据预测值和真实值之间的误差更新权重和偏置。在预测过程中,我们计算线性输出,然后使用激活函数将其转换为二进制输出。
最后,我们可以使用以下代码来训练和测试我们的模型:
```python
perceptron = Perceptron(learning_rate=0.1, n_iters=1000)
perceptron.fit(X, y)
y_pred = perceptron.predict(X)
print(y_pred)
```
这将打印出模型的预测结果。
希望这个例子对您有所帮助!
相关推荐
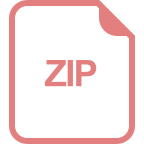












