用c#语言中的栈和队列判断字符串是否为回文
时间: 2023-12-25 08:28:53 浏览: 139
在C#语言中,我们可以使用栈(Stack)和队列(Queue)来判断字符串是否为回文。以下是一个简单的示例:
1. 首先,将字符串的每个字符依次压入栈中。
2. 然后,将栈中的字符依次弹出并与原字符串的对应位置进行比较。如果所有字符都相等,则说明该字符串是回文;否则,不是回文。
3. 接下来,将字符串的每个字符依次出队并添加到新的字符串中。
4. 最后,比较新字符串和原字符串是否相等。如果相等,则说明该字符串是回文;否则,不是回文。
以下是C#代码实现:
```csharp
using System;
using System.Collections.Generic;
class MainClass {
public static void Main (string[] args) {
string input = "level";
bool isPalindrome = IsPalindrome(input);
Console.WriteLine(isPalindrome); // 输出:True
}
public static bool IsPalindrome(string input) {
Stack<char> stack = new Stack<char>();
Queue<char> queue = new Queue<char>();
// 将字符串的每个字符依次压入栈中
for (int i = 0; i < input.Length; i++) {
stack.Push(input[i]);
}
// 将栈中的字符依次弹出并与原字符串的对应位置进行比较
for (int i = 0; i < input.Length; i++) {
char top = stack.Pop();
if (top != input[i]) {
return false;
}
}
// 将字符串的每个字符依次出队并添加到新的字符串中
string reversed = "";
while (stack.Count > 0) {
reversed += stack.Pop();
}
// 比较新字符串和原字符串是否相等
return reversed == new string(input.ToCharArray());
}
}
```
这段代码定义了一个名为`IsPalindrome`的方法,该方法接受一个字符串作为输入,并返回一个布尔值,表示该字符串是否为回文。在`Main`方法中,我们调用了`IsPalindrome`方法并输出结果。
阅读全文
相关推荐
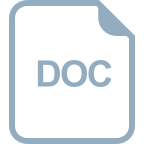
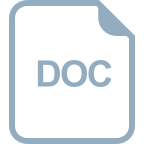
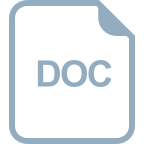
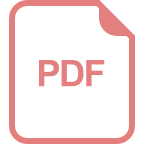

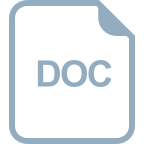
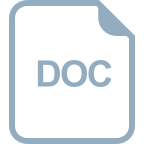
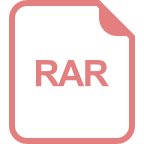
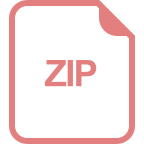
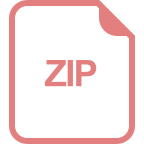
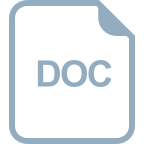
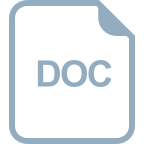
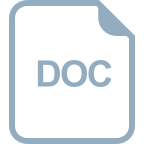
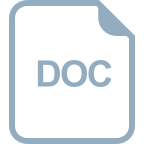
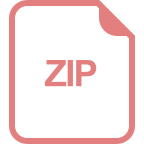
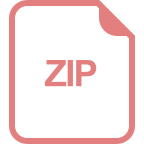
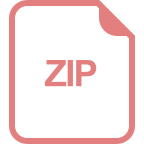