opencvsharp 计算LineSegmentPoint perpLine 与 LineSegmentPoint caliperLine 的交点
时间: 2024-02-03 13:12:49 浏览: 14
要计算LineSegmentPoint perpLine与LineSegmentPoint caliperLine的交点,你可以使用以下步骤:
1. 首先,计算出perpLine和caliperLine的斜率。如果斜率不存在,则表示这条线是竖直的,需要特殊处理。
2. 然后,计算出perpLine和caliperLine的截距。这可以通过将直线方程中的一个点代入来完成。
3. 接下来,计算perpLine和caliperLine的交点。你可以使用以下公式:x = (b2 - b1) / (m1 - m2),y = m1 * x + b1。
4. 最后,检查交点是否在两个线段之内。你可以使用点积和叉积来完成。
下面是示例代码,使用OpenCvSharp库计算出交点:
```csharp
using OpenCvSharp;
// 计算LineSegmentPoint perpLine与LineSegmentPoint caliperLine的交点
public Point2f CalculateIntersection(LineSegmentPoint perpLine, LineSegmentPoint caliperLine)
{
// 计算斜率
float m1 = (perpLine.P2.Y - perpLine.P1.Y) / (perpLine.P2.X - perpLine.P1.X);
float m2 = (caliperLine.P2.Y - caliperLine.P1.Y) / (caliperLine.P2.X - caliperLine.P1.X);
// 计算截距
float b1 = perpLine.P1.Y - m1 * perpLine.P1.X;
float b2 = caliperLine.P1.Y - m2 * caliperLine.P1.X;
// 计算交点
float x = (b2 - b1) / (m1 - m2);
float y = m1 * x + b1;
Point2f intersection = new Point2f(x, y);
// 检查交点是否在两个线段之内
if (IsPointOnLineSegment(intersection, perpLine) && IsPointOnLineSegment(intersection, caliperLine))
{
return intersection;
}
else
{
return new Point2f(-1, -1); // 交点不在两个线段之内
}
}
// 检查点是否在线段上
public bool IsPointOnLineSegment(Point2f point, LineSegmentPoint line)
{
float dotProduct = (point.X - line.P1.X) * (line.P2.X - line.P1.X) + (point.Y - line.P1.Y) * (line.P2.Y - line.P1.Y);
if (dotProduct < 0)
{
return false;
}
float lengthSquared = (line.P2.X - line.P1.X) * (line.P2.X - line.P1.X) + (line.P2.Y - line.P1.Y) * (line.P2.Y - line.P1.Y);
if (dotProduct > lengthSquared)
{
return false;
}
return true;
}
```
相关推荐
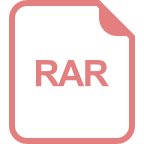
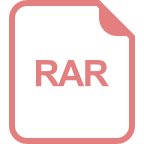














