爬取英雄联盟官网所有英雄信息(id,name,title,hp,mp) 并且把爬取的数据写入到csv文件。然后对爬取的数据进行处理 获取初始血量top10的英雄名称,用opts和Bar的方法以柱状图的形式进行可视化化显示(血量/名称)
时间: 2024-04-05 12:32:17 浏览: 17
好的,这是一个类似于上一个问题的任务,只是使用了不同的可视化库。以下是实现该任务的大致步骤:
1. 分析英雄联盟官网的页面结构,确定需要爬取的信息的所在位置和对应的HTML标签和属性。
2. 使用Python的爬虫库,如BeautifulSoup、Scrapy等,编写爬虫程序,从英雄联盟官网上获取英雄信息。
3. 将获取的英雄信息存储到CSV文件中,可以使用Python的CSV库来实现。
4. 使用Python的pandas库,读取CSV文件中的数据,并对数据进行处理,筛选出初始血量Top10的英雄。
5. 使用Python的pyecharts库,将筛选出的英雄的血量信息以柱状图的形式进行可视化。
下面是一个可能的实现代码,仅供参考:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
from pyecharts import options as opts
from pyecharts.charts import Bar
# 爬取英雄信息并存储到CSV文件中
def crawl_heroes():
url = 'https://www.leagueoflegends.com/zh-cn/champions/'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
heroes = []
for li in soup.find_all('li', class_='style__Wrapper-sc-12h96bu-0'):
hero_id = li['data-rg-id']
hero_name = li.find('span', class_='style__Text-sc-12h96bu-2').text.strip()
hero_title = li.find('span', class_='style__Title-sc-12h96bu-4').text.strip()
hero_hp = li.find('span', class_='style__StatValue-sc-12h96bu-5', text='血量').find_previous_sibling('span').text.strip()
hero_mp = li.find('span', class_='style__StatValue-sc-12h96bu-5', text='能量').find_previous_sibling('span').text.strip()
heroes.append({
'id': hero_id,
'name': hero_name,
'title': hero_title,
'hp': hero_hp,
'mp': hero_mp
})
df = pd.DataFrame(heroes)
df.to_csv('heroes.csv', index=False)
# 获取初始血量Top10的英雄
def get_top10_heroes():
df = pd.read_csv('heroes.csv')
df['hp'] = df['hp'].str.replace(',', '').astype('int')
top10 = df.sort_values(by='hp', ascending=False).head(10)
return top10
# 以柱状图的形式可视化英雄血量信息
def plot_heroes_hp(top10):
x_data = top10['name'].tolist()
y_data = top10['hp'].tolist()
bar = (
Bar()
.add_xaxis(x_data)
.add_yaxis("Initial HP", y_data)
.set_global_opts(
xaxis_opts=opts.AxisOpts(axislabel_opts=opts.LabelOpts(rotate=-45)),
title_opts=opts.TitleOpts(title="Top 10 Heroes by Initial HP"),
yaxis_opts=opts.AxisOpts(name="HP"),
)
)
bar.render("heroes_hp.html")
if __name__ == '__main__':
crawl_heroes()
top10 = get_top10_heroes()
print(top10)
plot_heroes_hp(top10)
```
这段代码中,我们首先定义了三个函数,分别是`crawl_heroes()`、`get_top10_heroes()`和`plot_heroes_hp()`。`crawl_heroes()`函数使用requests和BeautifulSoup库爬取英雄信息并存储到CSV文件中;`get_top10_heroes()`函数使用pandas库读取CSV文件中的数据,并筛选出初始血量Top10的英雄;`plot_heroes_hp()`函数使用pyecharts库将筛选出的英雄的血量信息以柱状图的形式进行可视化。在主函数中,我们依次调用这三个函数,实现了所需的功能。
相关推荐
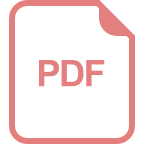
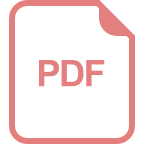
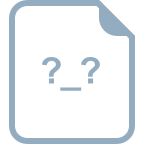
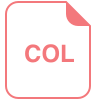
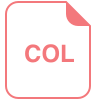
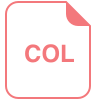
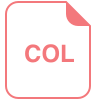
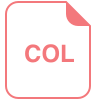









