用c++语言用单链表写一个多项式的乘法的代码
时间: 2024-10-16 07:10:15 浏览: 34
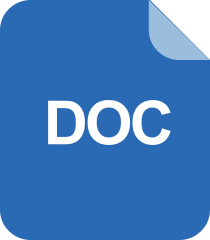
C++ 单链表实现多项式乘法
在C++中,我们可以使用单链表来表示多项式,并利用两个多项式的系数和指数信息来进行相乘操作。这里提供一个简单的示例,假设我们的多项式存储结构仅包含常数项和次数,以及指向下一个节点的指针:
```cpp
// 定义一个节点结构体
struct PolynomialTerm {
int coefficient; // 系数
int exponent; // 次数
PolynomialTerm* next; // 指向下一个节点的指针
};
// 创建一个新的多项式节点函数
PolynomialTerm* createTerm(int coefficient, int exponent) {
PolynomialTerm* term = new PolynomialTerm();
term->coefficient = coefficient;
term->exponent = exponent;
term->next = nullptr;
return term;
}
// 将两个多项式相乘的函数
void multiplyPolynomials(PolynomialTerm** result, PolynomialTerm* poly1, PolynomialTerm* poly2) {
*result = nullptr;
for (PolynomialTerm* p1 = poly1; p1 != nullptr; p1 = p1->next) {
for (PolynomialTerm* p2 = poly2; p2 != nullptr; p2 = p2->next) {
if (p1->exponent + p2->exponent > 0) { // 如果合并后的次数大于0
PolynomialTerm* temp = createTerm(p1->coefficient * p2->coefficient, p1->exponent + p2->exponent);
temp->next = (*result); // 更新结果链表
*result = temp;
}
}
}
}
// 示例使用
int main() {
PolynomialTerm* poly1 = createTerm(1, 2); // 1x^2
PolynomialTerm* poly2 = createTerm(2, 1); // 2x^1
PolynomialTerm* resultPoly = nullptr;
multiplyPolynomials(&resultPoly, poly1, poly2);
// 打印结果多项式(这里只是一个简化版本,实际可能需要额外处理)
while (resultPoly != nullptr) {
std::cout << resultPoly->coefficient << "x^" << resultPoly->exponent << " ";
resultPoly = resultPoly->next;
}
return 0;
}
```
阅读全文
相关推荐
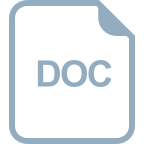
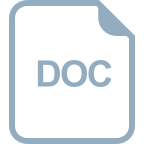
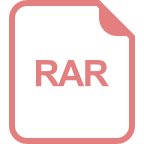
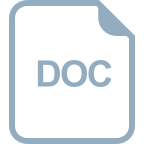








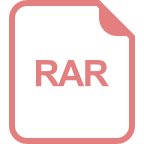
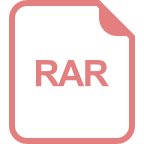
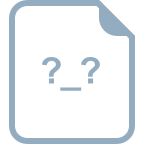
