c++用单链表实现多项式加减乘
时间: 2024-12-18 12:28:14 浏览: 6
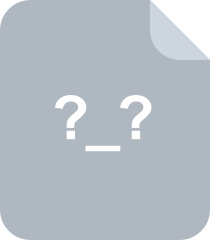
C++ 单链表实现多项式加减乘
在C++中,可以使用单链表来实现多项式的加、减和乘操作,因为每个节点通常代表多项式的系数和变量指数。以下是简单的步骤:
1. **定义节点结构**:
```cpp
struct Term {
int coefficient; // 系数
int exponent; // 指数
Term* next; // 指向下一个项的指针
};
```
2. **初始化链表**:
- 对于加法和减法,你可以创建两个链表分别存储要相加减的多项式。
- 对于乘法,需要额外的数据结构来存储中间结果,比如哈希表或树。
3. **加法**:
- 遍历两个链表,对于同次幂的项(即指数相同的),将系数相加,并更新链表节点。
```cpp
void addTerms(Term* &listA, Term* &listB) {
while (listA && listB) {
if (listA->exponent == listB->exponent) {
listA->coefficient += listB->coefficient;
if (listA->coefficient == 0) {
delete listA;
listA = listB;
} else {
listA = listA->next;
}
delete listB;
listB = listB->next;
} else if (listA->exponent < listB->exponent) {
listA = listA->next;
} else {
listB = listB->next;
}
}
// ...处理剩余项...
}
```
4. **减法**:
类似于加法,只需改变正负号判断即可。
5. **乘法**:
- 可以通过动态规划或递归遍历两个链表,对于每对项计算它们的积并插入到结果链表中。
```cpp
void multiplyTerms(Term* &result, const Term* &poly1, const Term* &poly2) {
for (Term* p1 = poly1; p1; p1 = p1->next) {
for (Term* p2 = poly2; p2; p2 = p2->next) {
result->coefficient += p1->coefficient * p2->coefficient;
result->exponent = p1->exponent + p2->exponent;
// ...分配新节点...
}
}
}
```
阅读全文
相关推荐
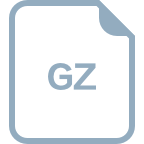
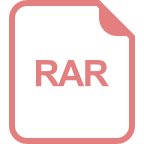




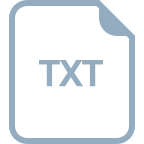
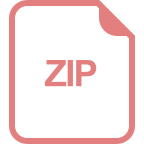
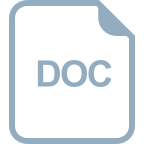
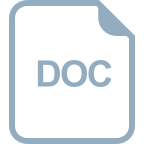
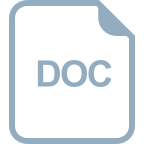

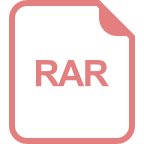
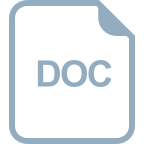
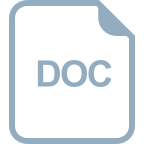
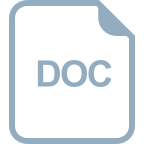