qqtablewidget在mousereleaseevent怎么获取鼠标所在的行号
时间: 2024-10-10 07:12:16 浏览: 33
QQTableWidget是一个基于Qt库的控件,它提供了一个表格式的用户界面。在`QTableWidget`中,处理鼠标释放事件通常涉及到获取事件的位置并映射到对应的行号。当你在一个单元格上释放鼠标,你可以通过以下步骤获取行号:
1. 首先,你需要注册一个`QTableWidget::cellClicked()`或`QTableWidget::cellDoubleClicked()`信号连接,因为`mouseReleaseEvent()`不会自动触发信号。
```cpp
connect(tableWidget, &QTableWidget::cellClicked, this, &YourClass::handleCellClick);
```
其中`YourClass::handleCellClick`是你自定义的槽函数。
2. 在槽函数`handleCellClick`中,你可以从`QMouseEvent`对象中提取出鼠标点击的列和行索引:
```cpp
void YourClass::handleCellClick(const QModelIndex& clickedIndex) {
int row = clickedIndex.row(); // 行号
// ...
}
```
`QModelIndex`是一个表示模型中数据项的引用,它的`.row()`方法返回行号。
相关问题
qqtablewidget重写mousereleaseevent怎么判断哪一行
QQTableWidget是一个基于Qt库的表格控件,用于显示数据并支持用户交互。如果你想在用户释放鼠标事件(`mouseReleaseEvent`)时确定点击的是哪一行,你需要捕获这个事件,并检查鼠标点击的位置对应于表格中的哪一行。
首先,在`QTableWidget`的子类中重写`mouseReleaseEvent(QMouseEvent *event)`方法:
```cpp
class CustomTableWidget : public QTableWidget {
public:
// 其他成员函数...
protected:
void mouseReleaseEvent(QMouseEvent *event) override {
int row = event->y() / height(); // 计算行索引,假设高度已知
int column = event->x() / width(); // 计算列索引,同样假设宽度已知
QModelIndex index = indexAt(column, row);
if (!index.isValid()) {
qDebug() << "Click outside the table";
} else {
int actualRow = index.row();
qDebug() << "Clicked on row: " << actualRow;
// 在这里你可以根据实际需要处理点击的行
}
QTableWidget::mouseReleaseEvent(event); // 一定要调用父类的方法,防止事件丢失
}
};
```
在这个例子中,我们将鼠标在屏幕上的Y坐标除以表格的高度来得到行号,X坐标除以宽度得到列号。然后我们获取点击位置对应的模型索引(`QModelIndex`),如果无效则表示点击了表格以外的地方,否则我们可以获取实际的行号。
mouseReleaseEvent
The mouseReleaseEvent is a signal in Qt that is emitted when a mouse button is released. This event is typically used to handle user interaction with graphical user interface (GUI) elements that respond to mouse clicks, such as buttons or sliders.
Here is an example of handling the mouseReleaseEvent in a custom Qt widget:
```
class MyWidget : public QWidget {
Q_OBJECT
public:
MyWidget(QWidget *parent = nullptr) : QWidget(parent) {}
protected:
void mouseReleaseEvent(QMouseEvent *event) override {
// handle the mouse release event
// event->pos() gives the position of the mouse cursor
// event->button() gives the button that was released
}
};
```
In this example, we override the mouseReleaseEvent function in our custom widget and add our own event handling code. The function receives a QMouseEvent object, which provides information about the mouse event, such as the position of the mouse cursor and the button that was released.
We can then use this information to perform some action, such as updating the state of our widget or triggering some other functionality.
阅读全文
相关推荐
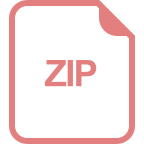
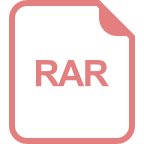
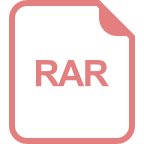














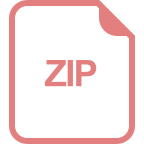