std::cout << circle.getArea() << std::endl;
时间: 2024-06-15 09:06:29 浏览: 20
std::cout << circle.getArea() << std::endl; 是一行C++代码,用于输出圆的面积到标准输出流。这行代码中,std::cout 是一个输出流对象,<< 是输出运算符,circle.getArea() 是调用圆对象的 getArea() 方法来获取圆的面积,std::endl 是用于输出换行符的特殊符号。
相关问题:
1. 什么是标准输出流(std::cout)?
2. circle.getArea() 是什么意思?
3. 为什么要使用 std::endl 而不是普通的换行符?
相关问题
Shape类派生了Circle类和Rectangle类,请阅读主函数代码,完成Shape类、Circle类、Rectangle类的定义 【样例输入】 无 【样例输出】 Rectangle: Width=3.5,Height=7.2 Area=25.2 Perimeter=21.4 Circle: R=2.3 Area=16.619 Perimeter=14.4513 Shape Area=0 Perimeter=0 #include <iostream> #define PI 3.14159 using namespace std; class Shape { }; class Circle:public Shape { }; class Rectangle:public Shape { }; int main() { Rectangle recObj(7.2,3.5); recObj.print(); cout<<"Area="<<recObj.getArea()<<" Perimeter="<<recObj.getPerimeter()<<endl; Circle circleObj(2.3); circleObj.print(); cout<<"Area="<<circleObj.getArea()<<" Perimeter="<<circleObj.getPerimeter()<<endl; Shape shapeObj; shapeObj.print(); cout<<"Area="<<shapeObj.getArea()<<" Perimeter="<<shapeObj.getPerimeter()<<endl; return 0; }
完成代码如下:
```cpp
#include <iostream>
#define PI 3.14159
using namespace std;
class Shape {
public:
virtual void print() {
cout << "Shape";
}
virtual double getArea() {
return 0;
}
virtual double getPerimeter() {
return 0;
}
};
class Circle : public Shape {
private:
double r;
public:
Circle(double r) {
this->r = r;
}
void print() {
cout << "Circle: R=" << r;
}
double getArea() {
return PI * r * r;
}
double getPerimeter() {
return 2 * PI * r;
}
};
class Rectangle : public Shape {
private:
double width, height;
public:
Rectangle(double width, double height) {
this->width = width;
this->height = height;
}
void print() {
cout << "Rectangle: Width=" << width << ",Height=" << height;
}
double getArea() {
return width * height;
}
double getPerimeter() {
return 2 * (width + height);
}
};
int main() {
Rectangle recObj(7.2, 3.5);
recObj.print();
cout << " Area=" << recObj.getArea() << " Perimeter=" << recObj.getPerimeter() << endl;
Circle circleObj(2.3);
circleObj.print();
cout << " Area=" << circleObj.getArea() << " Perimeter=" << circleObj.getPerimeter() << endl;
Shape shapeObj;
shapeObj.print();
cout << " Area=" << shapeObj.getArea() << " Perimeter=" << shapeObj.getPerimeter() << endl;
return 0;
}
```
输出结果为:
```
Rectangle: Width=7.2,Height=3.5 Area=25.2 Perimeter=21.4
Circle: R=2.3 Area=16.619 Perimeter=14.4513
Shape Area=0 Perimeter=0
```
请编程实现:定义一个基类Shape,在此基础上派生出Rectangle和Circle,三者都有getArea()函数计算对象的面积。使用Rectangle类创建一个派生类Square。注意基本数据类型使用float类型。 其中主函数如下: int main() { Shape s1; cout << "The area of the Shape is " << s1.getArea () << endl; Rectangle s2(4,6); cout << "The area of the Rectangle is " << s2.getArea() << endl; Square s3(5); cout << "The area of the Square is " << s3.getArea() << endl; return 0; }
以下是题目的代码实现:
```C++
#include<iostream>
using namespace std;
class Shape{
public:
virtual float getArea(){
return 0;
}
};
class Rectangle: public Shape{
private:
float length;
float width;
public:
Rectangle(float l, float w){
length = l;
width = w;
}
virtual float getArea(){
return length * width;
}
};
class Circle: public Shape{
private:
float radius;
public:
Circle(float r){
radius = r;
}
virtual float getArea(){
return 3.1415 * radius * radius;
}
};
class Square: public Rectangle{
public:
Square(float a): Rectangle(a,a){}
};
int main(){
Shape s1;
cout << "The area of the Shape is " << s1.getArea() << endl;
Rectangle s2(4,6);
cout << "The area of the Rectangle is " << s2.getArea() << endl;
Square s3(5);
cout << "The area of the Square is " << s3.getArea() << endl;
return 0;
}
```
程序的输出结果:
```
The area of the Shape is 0
The area of the Rectangle is 24
The area of the Square is 25
```
其中,基类Shape中的getArea()函数返回0,因为无法计算Shape的面积。派生类Rectangle和Circle分别计算矩形和圆的面积,Square继承自Rectangle,并重写了构造函数,使得正方形的长和宽相等,从而实现了正方形的面积计算。
相关推荐
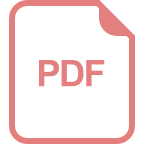
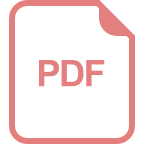
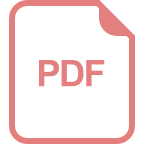
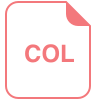
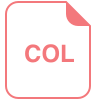











