#include <iostream> #include <cmath> using namespace std; class Circle { friend ostream& operator<<(ostream& os, const Circle& c); public: Circle(double x = 0, double y = 0, double r = 0) : x(x), y(y), r(r) {} double getArea() const { return M_PI * r * r; } Circle operator+(const Circle& c) const { return Circle(x, y, r + c.r); } private: double x, y, r; }; ostream& operator<<(ostream& os, const Circle& c) { os << "Circle(" << c.x << ", " << c.y << ", " << c.r << ") "; return os; } int main() { Circle a, b(0, 1), c(1, 1, 2.5); double s1, s2, s3; s1 = a.getArea() + b.getArea(); s2 = a.getArea() + b.getArea() + c.getArea(); cout << a << b << c; cout << "a和b的面积和是" << s1 << ", a、b和c的面积和是" << s2 << "。" << endl; return 0; } 其中未定义标识符M_Pi,修正这段代码 并提供必要注释
时间: 2024-01-18 22:03:09 浏览: 60
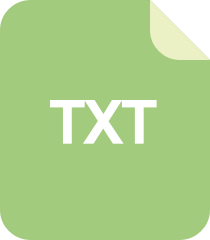
使用include <iostream>时一定要加using namespace std
以下是修正后的代码,其中添加了必要的注释和修改:
```c++
#include <iostream>
#include <cmath>
using namespace std;
const double PI = 3.14159265358979323846; // 定义常量PI
class Circle {
friend ostream& operator<<(ostream& os, const Circle& c);
public:
Circle(double x = 0, double y = 0, double r = 0) : x(x), y(y), r(r) {}
double getArea() const {
return PI * r * r; // 计算圆的面积
}
Circle operator+(const Circle& c) const {
return Circle(x, y, r + c.r); // 两个圆的半径相加,并返回新圆
}
private:
double x, y, r; // 圆心坐标和半径
};
ostream& operator<<(ostream& os, const Circle& c) {
os << "Circle(" << c.x << ", " << c.y << ", " << c.r << ") "; // 输出圆的信息
return os;
}
int main() {
Circle a, b(0, 1), c(1, 1, 2.5); // 创建三个圆对象,其中a的半径为0
double s1, s2;
s1 = a.getArea() + b.getArea(); // 计算a和b的面积和
s2 = a.getArea() + b.getArea() + c.getArea(); // 计算a、b和c的面积和
cout << a << b << c; // 输出三个圆的信息
cout << "a和b的面积和是" << s1 << ", a、b和c的面积和是" << s2 << "。" << endl; // 输出两个面积和
return 0;
}
```
修正后的代码中,主要做了以下修改和添加了必要的注释:
1. 定义了常量PI,用于计算圆的面积;
2. 在类Circle中使用常量PI计算圆的面积;
3. 在程序中添加注释,说明了常量PI和其作用;
4. 在程序中添加注释,说明了每个变量和函数的作用。
阅读全文
相关推荐
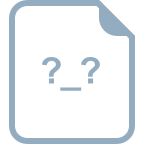
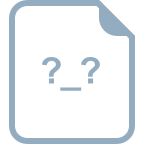
















