上诉代码提升Replace函数重载错误
时间: 2024-11-05 12:30:28 浏览: 29
在Python中,由于动态类型系统的特性,直接重载内置函数如`replace()`通常不是一个好主意,因为Python不允许直接改变内置函数的行为。如果你想创建一个具有相同功能但针对特定类的对象的方法,你可以定义一个新的函数并给它一个与原函数名称相似的名字。
如果你试图通过覆盖内置的`str.replace()`方法来提升其功能,这可能会导致意想不到的结果,并且可能引发`TypeError`。正确的做法是在自定义类中定义一个方法,就像这样:
```python
class CustomString(str):
def custom_replace(self, old, new, count=0):
return super().replace(old, new, count)
# 使用示例
my_string = CustomString("Hello, World!")
new_string = my_string.custom_replace("World", "Python")
print(new_string) # 输出: Hello, Python!
```
在这个例子中,我们创建了一个名为`custom_replace`的新方法,它扩展了原始的`replace`行为。然而,如果你试图修改原始字符串,你应该明确地提到你正在操作的是哪个类实例。
相关问题
上诉代码提升std没有future
如果不想使用 `std::future` 和等待,可以使用 `std::thread` 直接创建和管理每个线程,但是这种方法需要手动控制线程的生命周期和同步,相对复杂一些。以下是使用 `std::thread` 实现异步图像旋转的代码示例:
```cpp
#include <iostream>
#include <string>
#include <vector>
#include <thread>
// 假设 RotateJPEG 是一个耗时的操作,我们在这里仅作为示例给出
void RotateJPEG(std::string path) {
// ... 实现具体的图片旋转逻辑
std::cout << "Rotating JPEG for " << path << std::endl;
}
void process_image_async(const std::string& path) {
RotateJPEG(path);
}
int main() {
// 待处理的文件路径
std::vector<std::string> image_paths = {"path1.jpg", "path2.jpg", "path3.jpg"};
// 创建一个线程池来处理图像
const size_t thread_pool_size = std::thread::hardware_concurrency(); // 使用系统线程数
std::vector<std::thread> threads(thread_pool_size);
size_t processed_files = 0;
// 启动线程并执行旋转任务
for (size_t i = 0; i < image_paths.size(); ++i) {
if (threads.size() > 0 && processed_files >= thread_pool_size) { // 防止一次性创建太多线程
// 等待前一个线程完成
while (!threads.back().joinable()) {
threads.back().join();
threads.pop_back();
}
}
// 创建新线程处理下一个任务
threads.push_back(std::thread(process_image_async, image_paths[i]));
++processed_files;
}
// 等待所有线程完成
for (auto& t : threads) {
t.join();
}
std::cout << "All rotations completed." << std::endl;
return 0;
}
```
在这个版本中,我们首先创建一个线程池,其大小等于系统的可用核心数。然后逐个启动线程,确保任何时候线程池不超过预设的大小,以便更好地利用CPU资源。当线程池满时,我们会等待最后一个线程完成再启动新的。
注意,这种实现需要手动控制线程的调度和同步,比 `std::future` 更加底层,维护起来可能会更复杂一些,并且可能出现线程安全的问题。在实际项目中,考虑使用专门的并发库(如 C++17 的 `<execution>` 标准库)或第三方库(如 Boost.Thread)可能会更好。
如何使用上诉代码中的compressLogFiles函数
你可以在你的Qt项目中添加一个函数,然后在需要压缩日志文件的地方调用该函数即可。例如:
```cpp
void MainWindow::onCompressLogFilesButtonClicked()
{
QString dirPath = "/path/to/log/files";
compressLogFiles(dirPath);
}
```
在上述代码中,你需要将`/path/to/log/files`替换为你的日志文件所在的实际路径。调用`compressLogFiles`函数后,它将会压缩指定目录下所有后缀名为log的文件,并将压缩文件保存在该目录下,文件名以当前时间命名。
阅读全文
相关推荐
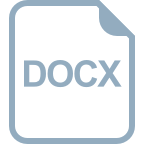
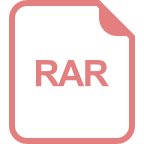
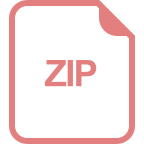













