c#CAD开发中列表如何同时存入Line和Polyline属性元素,再遍历此列表,判断列表内任意属性的两元素是否存在重合点,如果有则删除此两个元素输出新列表
时间: 2024-10-24 08:16:19 浏览: 22
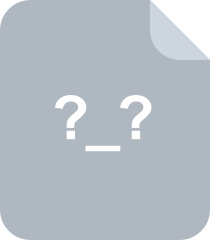
C#_CAD二次开发_快速选择dwg中的所有多段线Polyline和样条曲线Spline.cpp(两个函数)
在C# CAD开发中,如果你需要在一个列表(List<T>)中同时存储Line和Polyline对象,并遍历这个列表来判断线性元素之间的重叠,你可以这样做:
1. 定义一个`List<GeometryElement>`,其中`GeometryElement`是一个抽象类或接口,包含Line和Polyline这两种几何元素:
```csharp
public abstract class GeometryElement
{
public abstract bool HasOverlap(GeometryElement other);
}
public class Line : GeometryElement
{
// 线的定义...
}
public class Polyline : GeometryElement
{
// 多线段的定义...
}
```
2. 创建并初始化包含Line和Polyline对象的列表:
```csharp
List<GeometryElement> geometryList = new List<GeometryElement>();
geometryList.Add(new Line());
// 添加更多Line和Polyline实例...
geometryList.Add(new Polyline());
```
3. 遍历列表,检查每个元素与列表中其他元素是否有重叠:
```csharp
List<GeometryElement> newList = new List<GeometryElement>();
foreach (var element in geometryList)
{
if (element != null)
{
bool isDuplicateFound = false;
foreach (var existingElement in newList.Concat(geometryList.Where(e => e != element)))
{
if (existingElement.HasOverlap(element))
{
isDuplicateFound = true;
break;
}
}
if (!isDuplicateFound)
{
newList.Add(element);
}
}
}
```
在这个过程中,我们避免了直接比较`Line`和`Polyline`对象,而是通过它们共同的抽象基类`GeometryElement`来判断重叠。如果找到重复,则从`newList`中移除。
阅读全文
相关推荐

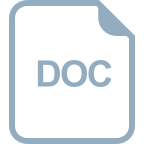
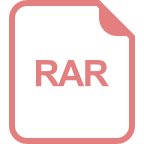












