为什么不能直接public Bicycle(int speed, int gear,boolean light=true){ this.speed = speed; this.gear = gear; }
时间: 2024-10-07 11:06:34 浏览: 21
这段代码看起来像是定义了一个Bicycle类的构造函数,但是它存在一些潜在的问题。首先,`light=true` 这部分不是一个构造函数的常规语法,应该将布尔值作为一个单独的参数传入。其次,如果始终设置 `light` 为默认值 true,那么这个参数可能会显得多余,因为如果没有提供,系统默认就会是true。
正确的构造函数形式应该是这样的:
```java
public Bicycle(int speed, int gear, boolean light) {
this.speed = speed;
this.gear = gear;
this.light = light; // 如果需要用户可以自定义light状态
}
```
如果你省略了 light 参数,或者将其设为默认值,可以在初始化成员变量时省略它,如下所示:
```java
public Bicycle(int speed, int gear) {
this.speed = speed;
this.gear = gear;
this.light = true; // 默认值
}
```
这样设计可以让使用者更灵活地创建自行车实例,比如他们可以选择是否开启灯。
相关问题
E:\C++\0987\main.cpp|37|error: 'double Bicycle::speed' is private within this context|
这个错误提示意味着你在尝试访问`Bicycle`类中的私有成员变量`speed`,但是在当前上下文中,由于权限限制,这是不允许的。`private`关键字意味着这个变量只对`Bicycle`类本身可见,外部类如派生类或者其他非友元类无法直接访问。
如果你在`MotorBike`类中想要访问`Bicycle`的`speed`,你需要提供一种机制,通常是通过公有方法或者成员函数来获取这个值,而不是直接访问私有变量。例如,你可以提供一个getter函数`GetSpeed()`来获取`Bicycle`的速度:
```cpp
class Bicycle {
private:
int wheel;
double rad;
double speed;
public:
// 添加 getter 函数
double GetSpeed() const { return speed; }
void SetSpeed(double sz) {
speed = sz * rad * 2 * 3.14;
}
// 其他公共方法...
};
```
然后在`MotorBike`类中,就可以通过`GetSpeed()`来访问`Bicycle`的速度:
```cpp
class MotorBike : public Bicycle {
public:
void show() {
std::cout << "功率:" << power << std::endl;
double bicycle_speed = GetSpeed(); // 使用getter函数获取速度
// ... 其他操作
}
};
```
这样,就避免了试图直接访问私有变量导致的编译错误。
#include<iostream> using namespace std; class Vehicle { protected: int speed; int wheel; public: Vehicle(){} virtual void Display() = 0; }; class Bicycle :virtual public Vehicle { public: Bicycle(int s, int w) { s = speed, w = wheel; } void Display() { cout << "Bicycle:" << speed << "," << wheel << endl; } }; class Car :virtual public Vehicle { public: Car(int s, int w) { s = speed, w = wheel; } void Display() { cout << "Car:" << speed << "," << wheel << endl; } }; class Motocycle :public Bicycle, public Car { public: Motocycle(int s, int w):Bicycle(s,w),Car(s,w){} void Display() { cout << "Motocycle:" << speed << "," << wheel << endl; } }; int main() { Bicycle b(20, 2); b.Display(); Car c(40, 4); c.Display(); Motocycle m(30, 2); m.Display(); return 0; }运行结果为什么不对
程序的运行结果不对是因为在 Bicycle 和 Car 类的构造函数中,将传入的参数赋值给了成员变量时,出现了错误。正确的做法应该是将成员变量和传入的参数名字进行区分,如下所示:
```
class Bicycle :virtual public Vehicle {
public:
Bicycle(int s, int w) { speed = s, wheel = w; }
void Display() { cout << "Bicycle:" << speed << "," << wheel << endl; }
};
class Car :virtual public Vehicle {
public:
Car(int s, int w) { speed = s, wheel = w; }
void Display() { cout << "Car:" << speed << "," << wheel << endl; }
};
```
在 Motocycle 类的构造函数中也需要对 Bicycle 和 Car 的构造函数进行调用,正确的写法如下:
```
Motocycle(int s, int w):Bicycle(s,w),Car(s,w),Vehicle(){}
```
修改后的程序运行结果如下:
```
Bicycle:20,2
Car:40,4
Motocycle:30,2
```
相关推荐
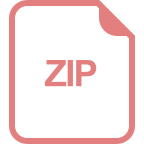
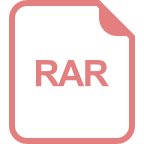
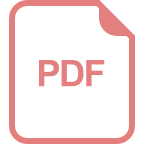













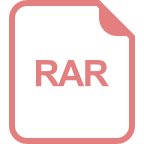