A星算法路径规划算法代码
时间: 2024-03-28 11:32:49 浏览: 68
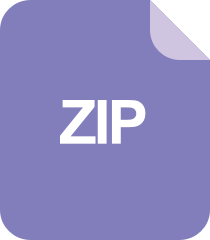
A星算法实现多机器人路径规划附matlab代码.zip

A星算法(A*)是一种常用的路径规算法,用于在图形或网络中找到最短路径。下面是A*算法的伪代码实现:
1. 初始化起始节点和目标节点。
2. 创建一个开放列表(open list)和一个关闭列表(closed list)。
3. 将起始节点添加到开放列表中。
4. 循环执行以下步骤直到找到目标节点或开放列表为空:
a. 从开放列表中选择具有最低估计总代价(f值)的节点作为当前节点。
b. 将当前节点从开放列表中移除,并将其添加到关闭列表中。
c. 对当前节点的相邻节点进行遍历:
- 如果相邻节点已经在关闭列表中,则忽略它。
- 如果相邻节点不在开放列表中,则将其添加到开放列表,并计算它的代价值(g值、h值和f值)。
- 如果相邻节点已经在开放列表中,比较当前路径是否更好(即更低的g值)。如果是,则更新相邻节点的父节点和代价值。
5. 如果开放列表为空,则表示无法找到路径。
6. 如果目标节点在关闭列表中,则从目标节点开始,通过父节点指针回溯路径直到起始节点。
下面是一个简单的A*算法的Python代码示例:
```python
class Node:
def __init__(self, x, y):
self.x = x
self.y = y
self.g = 0 # 从起始节点到当前节点的实际代价
self.h = 0 # 从当前节点到目标节点的估计代价
self.f = 0 # g值和h值的总和
self.parent = None # 当前节点的父节点
def astar(start, goal):
open_list = []
closed_list = []
open_list.append(start)
while open_list:
current_node = open_list[0]
current_index = 0
for index, node in enumerate(open_list):
if node.f < current_node.f:
current_node = node
current_index = index
open_list.pop(current_index)
closed_list.append(current_node)
if current_node == goal:
path = []
while current_node:
path.append((current_node.x, current_node.y))
current_node = current_node.parent
return path[::-1]
neighbors = get_neighbors(current_node) # 获取当前节点的相邻节点
for neighbor in neighbors:
if neighbor in closed_list:
continue
neighbor.g = current_node.g + get_distance(current_node, neighbor)
neighbor.h = get_distance(neighbor, goal)
neighbor.f = neighbor.g + neighbor.h
if neighbor in open_list:
if neighbor.g > current_node.g:
continue
else:
open_list.append(neighbor)
neighbor.parent = current_node
return None
# 示例函数,根据实际情况进行实现
def get_neighbors(node):
pass
# 示例函数,根据实际情况进行实现
def get_distance(node1, node2):
pass
# 示例用法
start_node = Node(0, 0)
goal_node = Node(5, 5)
path = astar(start_node, goal_node)
print(path)
```
阅读全文
相关推荐


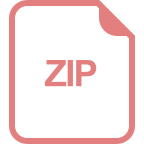
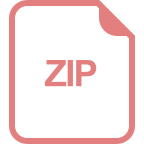
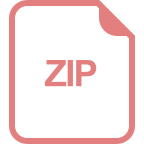
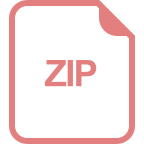
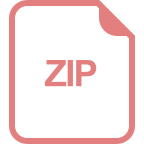
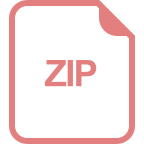
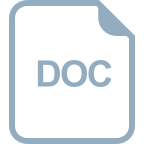
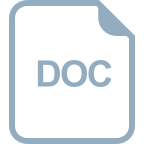



