用c语言写出如下 现有1个循环队列,队首到队尾的元素依次为8,3.2.9.5.6。约定T操作是 指队列中1个元素出队后再入队.Q操作是指队列中1个元素出队。则队列经过TITOTTQ 系列操作后,输出当前队列的元素。请完着以下程序
时间: 2024-10-27 22:03:02 浏览: 41
在C语言中,我们可以使用结构体`Queue`来表示循环队列,并包含两个数组`front`和`rear`分别用于存储队头和队尾元素的位置。同时需要一个整型变量`size`来表示队列的最大容量。下面是一个简单的实现:
```c
#include <stdio.h>
#define MAX_SIZE 10
typedef struct {
int data[MAX_SIZE];
int front, rear;
} Queue;
// 初始化循环队列
void init(Queue *q) {
q->front = -1;
q->rear = -1;
}
// 入队操作
void enqueue(Queue *q, int value) {
if (q->rear == (q->front + MAX_SIZE - 1) % MAX_SIZE) { // 队满
printf("Queue is full.\n");
return;
}
q->rear = (q->rear + 1) % MAX_SIZE; // 向后移动队尾指针
q->data[q->rear] = value;
if (q->front == -1) { // 如果队列为空,更新队头
q->front = q->rear;
}
}
// 出队操作
int dequeue(Queue *q) {
if (q->front == -1 || q->front == q->rear) { // 队列为空
printf("Queue is empty.\n");
return -1; // 返回错误码
}
int value = q->data[q->front]; // 获取并移除队头元素
q->front = (q->front + 1) % MAX_SIZE; // 更新队头指针
return value;
}
// 主函数演示TITOTTQ操作
int main() {
Queue q;
init(&q);
// 初始状态:8 3 2 9 5 6
enqueue(&q, 8);
enqueue(&q, 3);
enqueue(&q, 2);
enqueue(&q, 9);
enqueue(&q, 5);
enqueue(&q, 6);
for (int i = 0; i < 6; ++i) {
int operation = i % 2 ? 'T' : 'I';
switch (operation) {
case 'T': {
enqueue(&q, dequeue(&q));
break;
}
case 'I': {
int value = dequeue(&q);
enqueue(&q, value); // 操作后再次入队
break;
}
}
// 输出当前队列元素
if (q.front != -1) {
printf("%d ", q.data[q.front]);
} else {
printf("Queue is empty.\n");
}
}
return 0;
}
```
这个程序首先初始化了一个循环队列,然后按照"TITOTTQ"的操作顺序执行。注意在实际运行时,你需要根据输入的实际序列替换这一系列操作。
阅读全文
相关推荐
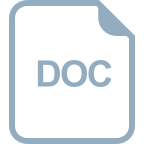
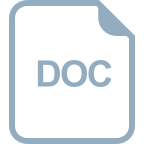
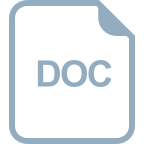















