用堆优化dijkstra算法求解最短路问题c++
时间: 2024-11-11 15:15:23 浏览: 18
Dijkstra算法是一种用于寻找图中两点之间最短路径的经典算法,通常用于有向无环图(DAG)和非负权重的图。在大规模数据处理中,尤其是涉及大量节点的情况,堆可以作为一个有效的优化工具来提高算法效率。
在C++中,我们可以使用优先队列(如`std::priority_queue`)来实现堆优化的Dijkstra算法。以下是基本步骤:
1. **初始化**:创建一个空的优先队列,并将起点放入队列,设置其距离为0,其余节点距离设为无穷大。
2. **主循环**:每次从队列中取出当前未访问的最小距离节点u。
- 对于u的所有邻居v,计算从起点到v的新距离(u的距离加上边(u, v)的权重)。如果新距离小于v当前已知距离,则更新v的距离并将其加入队列。
3. **检查结束条件**:当队列为空或者找到目标节点时,算法停止。
4. **结果返回**:返回终点的最短距离或路径信息。
这里的关键在于堆的高效插入和删除操作,它保证了每次取出的是当前距离最小的节点,从而避免了不必要的搜索。
```cpp
#include <queue>
#include <limits>
struct Node {
int dist;
int node_id;
bool operator<(const Node& other) const { return dist < other.dist; }
};
void dijkstra(int start, vector<vector<int>>& graph, vector<int>& shortest_distances) {
std::priority_queue<Node> pq;
pq.push({0, start});
shortest_distances[start] = 0;
while (!pq.empty()) {
int u = pq.top().node_id;
pq.pop();
for (int v : graph[u]) {
int new_dist = shortest_distances[u] + graph[u][v];
if (new_dist < shortest_distances[v]) {
shortest_distances[v] = new_dist;
pq.push({new_dist, v});
}
}
}
}
```
阅读全文
相关推荐
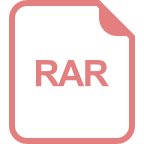
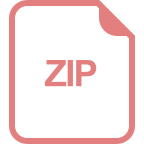
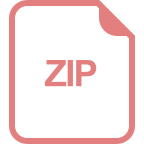
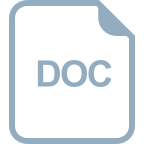
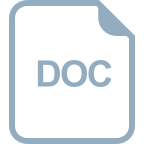

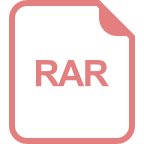
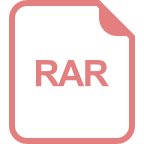
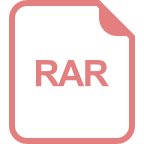
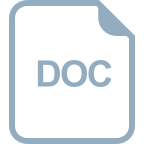
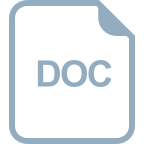
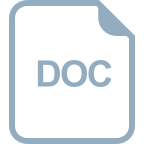


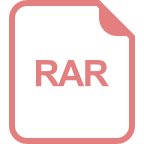
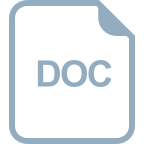
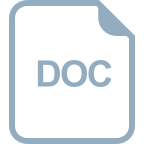
