char *p; int *p; double *p; sizeof(p) sizeof(*p) 看看大小是多少。
时间: 2023-08-16 07:09:51 浏览: 95
对于不同类型的指针,`sizeof(p)`返回指针本身的大小,而`sizeof(*p)`返回指针所指向的对象的大小。
1. 对于`char *p`,`sizeof(p)`通常是4或8(根据系统的位数而定),因为指针的大小是与系统位数相关的。
`sizeof(*p)`是1,因为`char`类型占用一个字节。
2. 对于`int *p`,`sizeof(p)`通常是4或8,同样取决于系统的位数。
`sizeof(*p)`通常是4,因为`int`类型占用4个字节。
3. 对于`double *p`,`sizeof(p)`通常是4或8,取决于系统的位数。
`sizeof(*p)`通常是8,因为`double`类型占用8个字节。
需要注意的是,指针的大小在不同系统和编译器上可能会有所不同。
相关问题
QT中将char*类型转为结构体
在 QT 中,可以使用 `QByteArray` 类或 `QDataStream` 类将 `char*` 类型的数据转换成结构体。
以下是一个示例代码,其中 `MyStruct` 是需要转换的结构体:
```c++
struct MyStruct {
int a;
double b;
};
// 创建一个 char* 类型的数据
char* charArray = new char[sizeof(MyStruct)];
MyStruct myStruct = {1, 2.0};
memcpy(charArray, &myStruct, sizeof(MyStruct));
// 使用 QByteArray 类将 char* 类型的数据转换成结构体
QByteArray byteArray(charArray, sizeof(MyStruct));
MyStruct newStruct;
memcpy(&newStruct, byteArray.constData(), sizeof(MyStruct));
// 使用 QDataStream 类将 char* 类型的数据转换成结构体
QDataStream in(byteArray);
MyStruct newStruct;
in.readRawData(reinterpret_cast<char*>(&newStruct), sizeof(MyStruct));
// 打印转换后的结构体成员变量值
qDebug() << newStruct.a << newStruct.b;
```
在上述代码中,首先创建了一个 `char*` 类型的数据,并将结构体数据拷贝到该数据中。然后,使用 `QByteArray` 类或 `QDataStream` 类将 `char*` 类型的数据转换成结构体。其中,使用 `QByteArray` 类时,可以直接使用 `constData()` 函数获得指向字节数组数据的指针;使用 `QDataStream` 类时,可以使用 `readRawData()` 函数将字节数组数据读取到结构体中。
需要注意的是,在进行转换时,需要确保字节数组的大小与结构体的大小相等。同时,如果使用 `QDataStream` 类进行转换,则需要使用 `reinterpret_cast` 进行类型转换。
udp char*转结构体
UDP协议是无连接的协议,传输的数据不同于TCP协议,UDP协议不会保证数据的可靠性和顺序性。因此,UDP数据包中的数据必须按照一定的格式进行打包和解包,即需要对数据进行结构体与字符数组之间的相互转换。
下面是一个示例代码,将一个结构体转换为字符数组:
```c++
struct MyStruct {
int a;
char b[10];
double c;
};
// 将结构体转换为字符数组
char* structToChar(MyStruct myStruct) {
char* data = new char[sizeof(MyStruct)];
memcpy(data, &myStruct, sizeof(MyStruct));
return data;
}
```
接下来是将字符数组转换为结构体的示例代码:
```c++
// 将字符数组转换为结构体
MyStruct charToStruct(char* data) {
MyStruct myStruct;
memcpy(&myStruct, data, sizeof(MyStruct));
return myStruct;
}
```
需要注意的是,在进行结构体与字符数组之间的转换时,必须保证两者的大小一致,否则会出现数据错误。同时,在进行网络传输时,还需要注意字节序的问题,因为不同的机器可能使用不同的字节序。可以使用网络字节序进行转换,常用的函数有`htonl()`和`ntohl()`等。
相关推荐
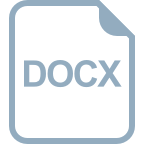
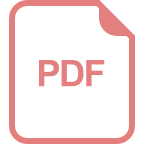
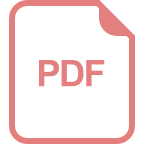












