P1067 [NOIP2009 普及组] 多项式输出 C语言
时间: 2024-10-13 08:16:56 浏览: 48
P1067 [NOIP2009 普及组] 的题目“多项式输出”通常涉及编程中处理数学中的多项式表达式,并将其转换成字符串形式输出。在C语言中,你可以通过动态内存分配和数组结构来存储多项式的系数及其对应的变量次数。
这里是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct {
int degree; // 多项式的最高次数
double* coefficients; // 存储各项的系数
} Polynomial;
// 动态创建一个多项式
Polynomial* create_polynomial(int n) {
Polynomial* poly = (Polynomial*)malloc(sizeof(Polynomial));
poly->degree = n;
poly->coefficients = (double*)malloc(n * sizeof(double)); // 初始化所有系数为0
for (int i = 0; i <= n; i++) {
poly->coefficients[i] = 0.0;
}
return poly;
}
// 输出多项式
void print_polynomial(const Polynomial* poly) {
if (poly->degree == 0) {
printf("0\n");
} else {
for (int i = poly->degree; i >= 0; i--) {
printf("%lf*x^%d + ", poly->coefficients[i], i);
}
printf("0\n"); // 移除最后一个加号
}
}
// 示例使用
int main() {
Polynomial* poly = create_polynomial(2); // 创建二次多项式
poly->coefficients[0] = 3.5; // a coefficient
poly->coefficients[1] = -2.0; // b coefficient
print_polynomial(poly); // 输出: "3.500000x^0 - 2.000000x^1 + 0.000000x^2"
free(poly->coefficients); // 释放内存
free(poly);
return 0;
}
```
阅读全文
相关推荐
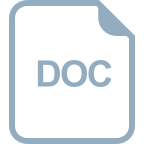
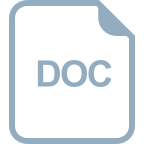
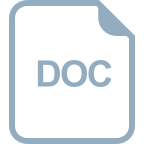
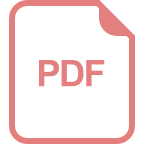

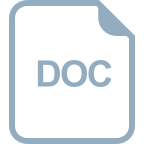
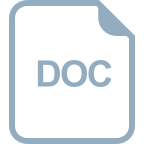
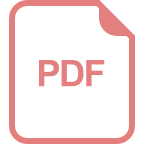
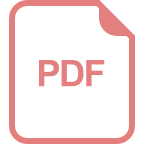
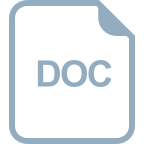


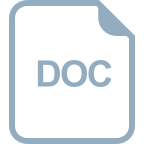
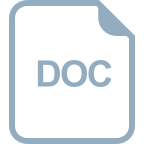
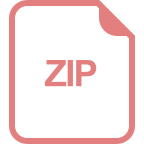
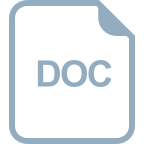
