torch.Tensor转为narray
时间: 2024-04-16 16:23:58 浏览: 15
要将torch.Tensor转换为numpy数组(narray),可以使用`.numpy()`方法。下面是一个示例:
```python
import torch
import numpy as np
# 创建一个torch.Tensor
tensor = torch.tensor([1, 2, 3])
# 将torch.Tensor转换为numpy数组
narray = tensor.numpy()
print(narray)
```
输出:
```
[1 2 3]
```
注意,在转换为narray之前,请确保Tensor位于CPU上,因为numpy不支持GPU上的张量。如果你的Tensor在GPU上,可以使用`.cpu()`方法将其移动到CPU上,然后再进行转换:
```python
narray = tensor.cpu().numpy()
```
这样就可以将torch.Tensor成功地转换为numpy数组。
相关问题
np.ndarray转为torch.tensor
将np.ndarray转换为torch.tensor可以使用torch.from_numpy()函数。具体操作如下:
```python
import numpy as np
import torch
# 创建一个numpy数组
arr = np.array([[1, 2, 3], [4, 5, 6]])
# 将numpy数组转换为torch.tensor
tensor = torch.from_numpy(arr)
print(tensor)
```
输出结果为:
```
tensor([[1, 2, 3],
[4, 5, 6]], dtype=torch.int32)
```
如果将一个numpy.array型的图像转为torch.tensor型
的图像,应该怎么做?
可以使用torch.from_numpy()函数将numpy.array型的图像转为torch.tensor型的图像。具体代码如下:
import torch
import numpy as np
# 假设img是一个numpy.array型的图像
img = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# 将img转为torch.tensor型的图像
img_tensor = torch.from_numpy(img)
print(img_tensor)
输出结果为:
tensor([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]], dtype=torch.int32)
相关推荐
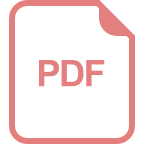
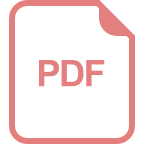
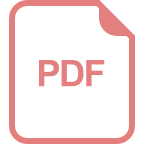













