torch.Size([3, 704, 704])怎么转为Numpy数据
时间: 2024-05-14 20:12:45 浏览: 52
可以使用以下代码将PyTorch张量转换为NumPy数组:
```python
import numpy as np
import torch
tensor = torch.randn(3, 704, 704) # 一个示例张量
numpy_array = tensor.cpu().detach().numpy() # 转换为NumPy数组
print(numpy_array.shape) # 输出NumPy数组的形状
```
其中,`detach()`方法用于从计算图中分离张量,以避免梯度计算,`cpu()`方法用于将张量传输到CPU,因为NumPy数组不支持GPU。最后,使用`numpy()`方法将张量转换为NumPy数组。
相关问题
def __call__(self, Image, data, index=None): Image.requires_grad_(True) # 将输入设置为需要计算梯度 data.requires_grad_(True) self.model.requires_grad_(True) # self.model.classifier.requires_grad_(True) output = self.forward_static(Image.cuda(), data.cuda()) if index is None: index = np.argmax(output.cpu().data.numpy()) one_hot = np.zeros((1, output.size()[-1]), dtype=np.float32) one_hot[0][index] = 1 one_hot = Variable(torch.from_numpy(one_hot), requires_grad=True) if self.cuda: one_hot = torch.sum(one_hot.cuda() * output) else: one_hot = torch.sum(one_hot * output) one_hot.backward() aa = input.grad output = input.grad.cpu().data.numpy() output = output[0, :, :, :] return output 代码中如何让整个模型参与梯度计算
在代码中,通过以下语句将整个模型设置为需要计算梯度:
```python
self.model.requires_grad_(True)
```
这将使整个模型中的参数都能够参与梯度计算。同时,也可以将模型中的某些特定层设置为需要计算梯度,例如:
```python
self.model.classifier.requires_grad_(True)
```
这将使模型中的分类器层的参数能够参与梯度计算。需要注意的是,为了正确计算梯度,输入的图像和数据也需要设置为需要计算梯度。
train_x_tensor = torch.from_numpy(train_x).type(torch.FloatTensor).to(DEVICE) # (B, N, F, T) train_target_tensor = torch.from_numpy(train_target).type(torch.FloatTensor).to(DEVICE) # (B, N, T) train_dataset = torch.utils.data.TensorDataset(train_x_tensor, train_target_tensor) train_loader = torch.utils.data.DataLoader(train_dataset, batch_size=batch_size, shuffle=shuffle)是什么意思
这段代码是将 numpy 数组转换为 PyTorch 的张量,并将其放置在 GPU 上进行训练。具体来说,它完成以下操作:
- 将训练数据集(train_x 和 train_target)转换为 PyTorch 的张量。
- 将张量类型转换为 FloatTensor(在 PyTorch 中,FloatTensor 是默认的张量类型)。
- 将张量放置在指定的设备(DEVICE)上,这里通常指的是 GPU。
- 使用 PyTorch 的 TensorDataset 类将训练数据集打包成一个数据集对象。
- 使用 PyTorch 的 DataLoader 类将数据集对象转换为数据加载器,用于批量加载训练数据。batch_size 参数指定了每个批次的大小,shuffle 参数指定了是否需要对数据进行随机重排。
阅读全文
相关推荐
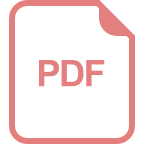
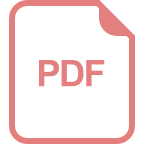














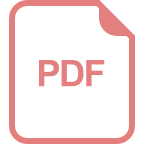