class GameBoard: def __init__(self, cell_width,margin,n,screen): self.n = n self.margin = margin self.cell_width = cell_width self.screen = screen self.screen.fill(Color.ORANGE) self.draw_board() self.draw_buttons() def draw_board(self): for i in range(self.n): pygame.draw.line(self.screen,Color.BLACK, (self. margin,self.margin + self.cell_width*i), (self.margin + (self.n-1)*self.cell_width,self.margin + self.cell_width*i), 2) for i in range(self.n): pygame.draw.line(self.screen, Color.BLACK, (self.margin + self.cell_width * i,self.margin), (self.margin + self.cell_width * i,self.margin + (self.n - 1) * self.cell_width), 2) def draw_buttons(self): pygame.draw.rect(self.screen, Color.BLACK, [self.margin + self.margin + self.cell_width * (self.n - 1) + 5, 50, 100, 50], 1) font = pygame.font.SysFont('宋体',30) txt = font.render('QUIT',True, Color.BLACK) self.screen.blit(txt, (self.margin + self.cell_width * (self.n - 1) + 45, 65)) pygame.draw.rect(self.screen, Color.BLACK, [self.margin + self.margin + self.cell_width * (self.n - 1) + 5, 350, 100, 50], 1) font = pygame.font.SysFont('宋体', 30) txt = font.render('Restart', True, Color.BLACK) self.screen.blit(txt, (self.margin + self.cell_width * (self.n - 1) + 45, 365)) def draw_first_chess(self): x,y = 610,410 pygame.draw.circle(self.screen,Color.BLACK,(x,y),self.cell_width // 2-2) def drawchess(self,row,col,color): x,y = col * self.cell_width +self.margin,row*self.cell_width + self.margin if color == 1: pygame.draw.circle(self.screen,Color.BLACK,(x,y),self.cell_width//2 - 1) else: pygame.draw.circle(self.screen, Color.WHITE, (x, y), self.cell_width // 2 - 1) def draw_now_chess(self,color): x,y = 500,1000 if color == 1: pygame.draw.circle(self.screen,Color.BLACK,(x,y),self.cell_width//2-2) else: pygame.draw.circle(self.screen,Color.BLACK,(x,y),self.cell_width//2-2) def draw_box(self,txt): pygame.draw.rect(self.screen,Color.RED, [150,175,400,100],1) font = pygame.font.SysFont('宋体', 80) txt_obj = font.render(txt, True, Color.RED) self.screen.blit(txt_obj, (200, 200))
时间: 2024-02-14 22:27:35 浏览: 248
这段代码是一个名为GameBoard的类,用于绘制一个游戏板。它有几个方法:
1. __init__方法是类的构造函数,它接受cell_width、margin、n和screen等参数,并初始化成员变量。它还调用draw_board和draw_buttons方法来绘制游戏板和按钮。
2. draw_board方法用于绘制游戏板的网格线。
3. draw_buttons方法用于绘制游戏板上的按钮,包括QUIT和Restart。
4. draw_first_chess方法用于绘制首个棋子。
5. drawchess方法用于根据给定的行、列和颜色绘制棋子。
6. draw_now_chess方法用于绘制当前棋子。
7. draw_box方法用于绘制一个红色边框的文本框。
该类使用了pygame库来进行绘图操作。
阅读全文
相关推荐
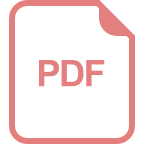
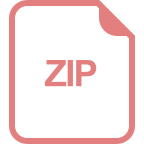
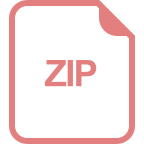
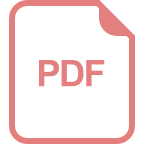
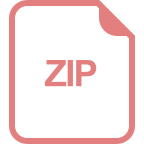
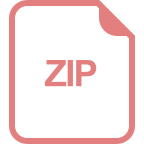
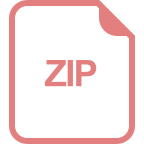
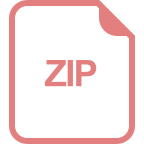
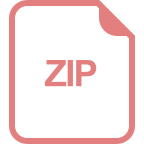
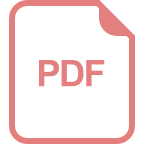
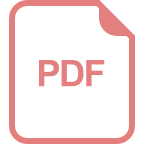
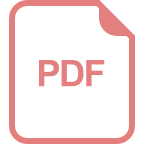
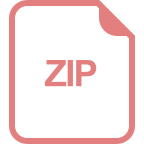
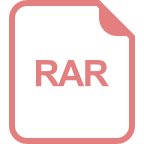
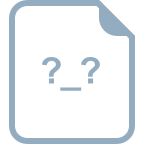
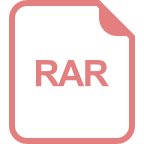
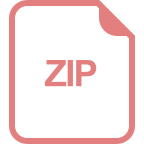
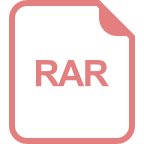
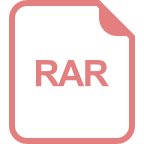