利用C#,帮我给出一个一个矩形知道中心坐标、长度、宽度,旋转a度后,四个点的坐标
时间: 2024-10-01 13:03:16 浏览: 62
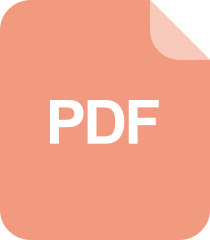
halcon拓展系列—计算任意矩形的四个角点坐标
在C#中,我们可以创建一个名为`RectangleRotation`的类,该类包含矩形的中心坐标、长度和宽度属性,并提供一个方法`Rotate`用于计算旋转后的四点坐标。这里我们假设旋转是围绕中心点进行的。
```csharp
using System;
public class RectangleRotation
{
// 矩形中心坐标
public Point Center { get; set; }
// 长度和宽度
public double Length { get; set; }
public double Width { get; set; }
// 旋转角度(弧度)
private double rotationAngleInRadians;
public RectangleRotation(Point center, double length, double width, double angleInDegrees)
{
Center = center;
Length = length;
Width = width;
rotationAngleInRadians = Math.PI * (angleInDegrees / 180);
}
// 计算旋转后的四个顶点坐标
public void Rotate()
{
double newX = Center.X + Length / 2 * (Math.Cos(rotationAngleInRadians) - (-Math.Cos(rotationAngleInRadians)));
double newY = Center.Y + Length / 2 * (Math.Sin(rotationAngleInRadians) - (-Math.Sin(rotationAngleInRadians)));
// 左上角
Point topLeft = new Point(newX - Width / 2, newY - Width / 2);
// 右上角
Point topRight = new Point(newX + Width / 2, newY - Width / 2);
// 左下角
Point bottomLeft = new Point(newX - Width / 2, newY + Width / 2);
// 右下角
Point bottomRight = new Point(newX + Width / 2, newY + Width / 2);
// 存储结果
TopLeft = topLeft;
TopRight = topRight;
BottomLeft = bottomLeft;
BottomRight = bottomRight;
}
// 这里只是示例,实际应用中可能会有TopLeft等变量存储旋转后的坐标
public Point TopLeft { get; private set; }
public Point TopRight { get; private set; }
public Point BottomLeft { get; private set; }
public Point BottomRight { get; private set; }
// 示例如何使用
public static void Main(string[] args)
{
Point center = new Point(50, 50); // 中心坐标
double length = 100; // 长度
double width = 75; // 宽度
double angle = 45; // 旋转角度
var rectangle = new RectangleRotation(center, length, width, angle);
rectangle.Rotate();
Console.WriteLine($"旋转后左上角 ({rectangle.TopLeft.X}, {rectangle.TopLeft.Y}) 右上角 ({rectangle.TopRight.X}, {rectangle.TopRight.Y})");
// ... 继续打印其他两个点
}
}
```
阅读全文
相关推荐
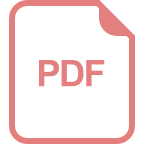
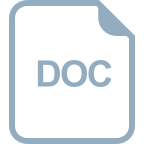















