使用c#实现输入四个点坐标拟合最大外接矩形,输出最小外接矩形中心点的坐标,不要用OpenCV
时间: 2024-02-23 20:03:18 浏览: 18
首先,我们可以使用C#中的Point类来表示点的坐标,代码如下:
```csharp
class Point
{
public int x;
public int y;
public Point(int x, int y)
{
this.x = x;
this.y = y;
}
}
```
然后,我们可以编写一个函数来计算两点之间的距离:
```csharp
double Distance(Point p1, Point p2)
{
return Math.Sqrt(Math.Pow(p1.x - p2.x, 2) + Math.Pow(p1.y - p2.y, 2));
}
```
接下来,我们可以编写一个函数来计算一个矩形的面积:
```csharp
double RectangleArea(Point p1, Point p2, Point p3, Point p4)
{
double d1 = Distance(p1, p2);
double d2 = Distance(p2, p3);
double d3 = Distance(p3, p4);
double d4 = Distance(p4, p1);
double diagonal1 = Distance(p1, p3);
double diagonal2 = Distance(p2, p4);
double s1 = (d1 + d3 + diagonal1) / 2;
double s2 = (d2 + d4 + diagonal2) / 2;
double area1 = Math.Sqrt(s1 * (s1 - d1) * (s1 - d3) * (s1 - diagonal1));
double area2 = Math.Sqrt(s2 * (s2 - d2) * (s2 - d4) * (s2 - diagonal2));
return area1 + area2;
}
```
接下来,我们可以编写一个函数来计算一个矩形的中心点坐标:
```csharp
Point RectangleCenter(Point p1, Point p2, Point p3, Point p4)
{
int centerX = (p1.x + p2.x + p3.x + p4.x) / 4;
int centerY = (p1.y + p2.y + p3.y + p4.y) / 4;
return new Point(centerX, centerY);
}
```
最后,我们可以编写一个函数来实现拟合最大外接矩形的功能:
```csharp
Point FitRectangle(Point p1, Point p2, Point p3, Point p4)
{
Point[] points = new Point[] { p1, p2, p3, p4 };
double maxArea = 0;
Point maxCenter = null;
for (int i = 0; i < 4; i++)
{
Point p = points[i];
Point[] otherPoints = points.Where(point => point != p).ToArray();
for (int j = 0; j < 3; j++)
{
Point p2 = otherPoints[j];
Point[] otherPoints2 = otherPoints.Where(point => point != p2).ToArray();
for (int k = 0; k < 2; k++)
{
Point p3 = otherPoints2[k];
Point p4 = otherPoints2.Where(point => point != p3).First();
double area = RectangleArea(p, p2, p3, p4);
if (area > maxArea)
{
maxArea = area;
maxCenter = RectangleCenter(p, p2, p3, p4);
}
}
}
}
return maxCenter;
}
```
这个函数的实现原理是,枚举所有可能的矩形,计算它们的面积,找到面积最大的矩形,并计算它的中心点坐标。
相关推荐
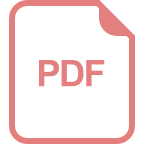














