DBSCAN算法c++实现
时间: 2023-11-25 07:46:58 浏览: 53
DBSCAN算法的C++实现需要以下步骤:
1. 定义一个Point类,用于存储每个点的坐标和聚类信息。
2. 定义一个DBSCAN类,包含以下成员函数:
- init函数:初始化N个坐标点。
- regionQuery函数:计算每个点的邻域内的点。
- expandCluster函数:扩展聚类。
- dbscan函数:执行DBSCAN算法。
3. 在main函数中,创建一个DBSCAN对象并调用dbscan函数进行聚类。
下面是一个简单的DBSCAN算法C++实现的代码示例:
```
#include <iostream>
#include <cstdlib>
#include <ctime>
#include <vector>
#include <cmath>
using namespace std;
const int N = 100; // 坐标点数量
const double eps = 1.0; // 邻域半径
const int minPts = 5; // 最小邻域点数
class Point {
public:
double x, y;
int cluster;
};
class DBSCAN {
public:
Point point[N];
vector<int> neighborPts[N];
void init(int n) {
srand((unsigned)time(NULL));
for(int i=0; i<n; i++) {
point[i].x = rand() % (N+1);
point[i].y = rand() % (N+1);
point[i].cluster = -1;
}
}
void regionQuery(int p) {
neighborPts[p].clear();
for(int i=0; i<N; i++) {
if(i == p) continue;
double dist = sqrt(pow(point[i].x - point[p].x,2) + pow(point[i].y - point[p].y, 2));
if(dist <= eps) {
neighborPts[p].push_back(i);
}
}
}
void expandCluster(int p, int c) {
point[p].cluster = c;
for(int i=0; i<neighborPts[p].size(); i++) {
int np = neighborPts[p][i];
if(point[np].cluster == -1) {
point[np].cluster = c;
regionQuery(np);
if(neighborPts[np].size() >= minPts) {
expandCluster(np, c);
}
}
}
}
void dbscan() {
int c = 0;
for(int i=0; i<N; i++) {
if(point[i].cluster != -1) continue;
regionQuery(i);
if(neighborPts[i].size() < minPts) {
point[i].cluster = 0; // 噪声点
} else {
c++;
expandCluster(i, c);
}
}
}
};
int main() {
DBSCAN dbscan;
dbscan.init(N);
dbscan.dbscan();
for(int i=0; i<N; i++) {
cout << "Point " << i << ": (" << dbscan.point[i].x << ", " << dbscan.point[i].y << ") Cluster: " << dbscan.point[i].cluster << endl;
}
return 0;
}
```
相关推荐
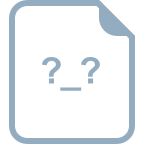














