使用【c】写【HOG特征提取不使用opencv】的代码
时间: 2024-09-26 18:10:43 浏览: 41
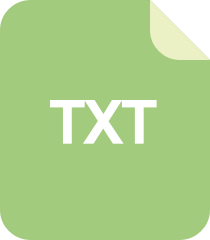
hog特征提取,c版本代码

霍夫关键点检测(Histogram of Oriented Gradients, HOG)是一种用于计算机视觉中的特征描述符,常用于行人检测、物体识别等场景。虽然OpenCV提供了一个方便的接口来进行HOG特征提取,但如果你想不使用OpenCV自己编写HOG特征提取算法,以下是一个简单的步骤和伪代码概述:
1. **计算梯度**:首先,你需要计算图像的灰度值以及每个像素点周围的梯度方向和大小。
```c
// 假设img为RGB图像,我们先转换成灰度
int gray_img[height][width];
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
// 取R、G、B三个分量的平均作为灰度值
gray_img[y][x] = (img[y][x][0] + img[y][x][1] + img[y][x][2]) / 3;
}
}
```
2. **计算梯度直方图**:对于每个局部窗口(cell),统计该区域内梯度方向的分布,形成一个直方图。
```c
// 定义窗口尺寸和步长
const int cell_size = ...;
const int block_stride = ...;
// 初始化HOG数组
float hog[block_height][block_width][orientation_bins];
for (int block_y = 0; block_y < block_height; block_y += block_stride) {
for (int block_x = 0; block_x < block_width; block_x += block_stride) {
for (int y = block_y; y < block_y + cell_size && y < height; ++y) {
for (int x = block_x; x < block_x + cell_size && x < width; ++x) {
// 计算梯度方向
float grad_dir = atan2(gray_img[y+1][x] - gray_img[y][x], gray_img[y][x+1] - gray_img[y][x]);
// 将角度映射到预定义的区间
int orientation_index = (grad_dir * (orientation_bins - 1)) / (M_PI * 2);
// 更新直方图
hog[block_y/block_stride][block_x/block_stride][orientation_index]++;
}
}
}
}
```
3. **归一化**:对直方图进行归一化,通常采用L2范数。
```c
// 对每一行(block)的直方图进行归一化
for (int i = 0; i < block_height*block_width; ++i) {
float sum = 0;
for (int j = 0; j < orientation_bins; ++j) {
sum += hog[i][j];
}
for (int j = 0; j < orientation_bins; ++j) {
hog[i][j] /= sum;
}
}
```
4. **计算描述子**:将所有块的直方图拼接起来形成最终的HOG描述子。
```c
// 最后,把整个block的HOG描述子连接起来
unsigned char hog_descriptor[block_height*block_width*orientation_bins];
memcpy(hog_descriptor, hog, sizeof(float) * block_height*block_width*orientation_bins);
```
请注意,这只是一个简化的版本,实际的HOG算法可能包括更复杂的细节,如滑动窗口、空间金字塔等,以提高性能和鲁棒性。此外,这个实现并没有包含非极大值抑制(NMS)和其他关键优化。
阅读全文
相关推荐
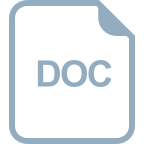
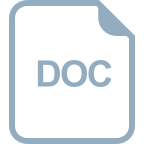

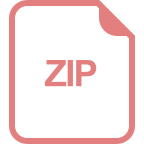
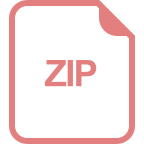
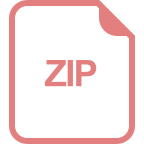
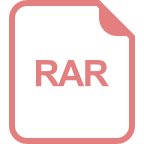
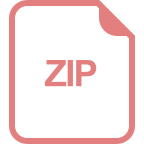
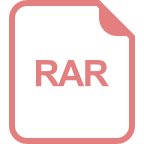
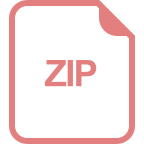
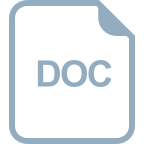
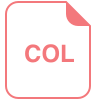




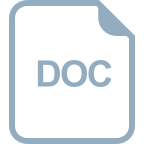
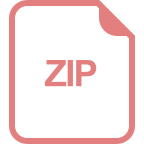