如何使用Python编程语言实现多项式的乘法运算?
时间: 2024-11-09 19:31:33 浏览: 17
在Python中,你可以使用内建的`functools.reduce()`函数结合列表推导式或者直接使用循环来实现两个多项式的乘法。这里我会展示一种使用`reduce()`和列表推导式的方法:
```python
from functools import reduce
# 定义多项式类
class Polynomial:
def __init__(self, coefficients):
self.coefficients = coefficients
# 多项式乘法的核心计算
@staticmethod
def multiply_coefficients(coeff1, coeff2, power):
return coeff1 * coeff2, power + 1
def multiply(self, other):
result = [0] * (len(self.coefficients) + len(other.coefficients) - 1)
for i in range(len(self)):
for j in range(len(other)):
new_coefficient, combined_power = Polynomial.multiply_coefficients(
self.coefficients[i], other[j], i + j
)
result[combined_power - 1] += new_coefficient
return Polynomial(result)
# 示例:创建两个多项式并相乘
poly1 = Polynomial([1, 2, 3])
poly2 = Polynomial([4, 5])
result = poly1.multiply(poly2)
```
在这个例子中,我们首先创建了`Polynomial`类,其中包含了系数列表。然后定义了一个静态方法`multiply_coefficients`用于计算新系数和组合的幂次。`multiply`方法则遍历两个多项式的系数,并根据乘法规则累加结果。
阅读全文
相关推荐
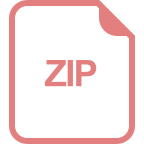
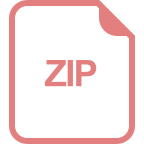
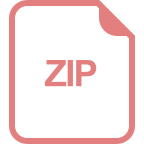













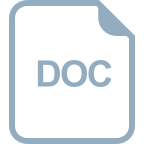
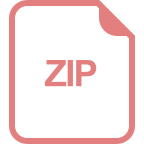
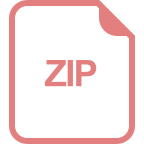